Hi dudes…!!! Here I’m coming with a new tutorial. We have already seen lots of tutorials using LCD. If you want to see those tutorials please click here. In previous LCD tutorials, we were used that LCD in 8-bit mode. But here we will see LCD 4-bit interfacing with 8051 Microcontroller. Okay, can you tell me what is 8 bit mode and 4 bit mode? Any guess. Well.
Table of Contents
LCD 4-bit interfacing with 8051 Microcontroller
Introduction
8-bit mode – Using 8 data lines in LCD (totally 8 data lines are there)
4-bit mode – Using only 4 data lines in the LCD module
8-bit mode is already working and that looks awesome. Then why we are going to 4-bit mode? This is the question that comes to everyone’s mind whenever I said 4-bit mode. Yeah, that 8-bit mode is nice. But but but… Just assume. I’m doing one project which requires more hardware. But 8051 have only 32 GPIOs. So in that time I can use this 4-bit mode and reduce the pin required for the LCD module. Am I right? Great. That’s why 4-bit mode also important. Already we know the LED’s operation. If we want to enable 4-bit mode we have to do small modifications in the normal method. Let’s see that.
In initializing time we have to give 0x28 command. That’s all.
|
|
LCD Initializing
void lcd_init() { cmd(0x02); cmd(0x28); cmd(0x0c); cmd(0x06); cmd(0x8f); }
Sending command
Here everything is the same except the way of data writing. Here we have only 4 bits. So we need to send nibble by nibble. So first we need to send first nibble then followed by the second. See that code. I’m writing into Port 2’s last 4 bits. Because the last 4 bits are connected to LCD.
void cmd(unsigned char a) { rs=0; rw=0; P2&=0x0F; //clearing the last 4 bits without disturbing first 4 bits P2|=(a&0xf0); //writing higher nibble e=1; delay(); e=0; delay(); P2&=0x0f; //clearing the last 4 bits without disturbing first 4 bits P2|=(a<<4&0xf0); //writing lower nibble e=1; delay(); e=0; delay(); }
Sending Data
Same as sending data.
void dat(unsigned char b) { rs=1; rw=0; P2&=0x0F; //clearing the last 4 bits without disturbing first 4 bits P2|=(b&0xf0); //writing higher nibble e=1; delay(); e=0; delay(); P2&=0x0f; //clearing the last 4 bits without disturbing first 4 bits P2|=(b<<4&0xf0); //writing lower nibble e=1; delay(); e=0; delay(); }
PIN DIAGRAM
RS is connected to P2.0
RW is connected to P2.1
|
|
EN is connected to P2.2
4 data lines are connected into P2. 4 to P2.7
CODE
#include<reg51.h> sbit rs=P2^0; sbit rw=P2^1; sbit e=P2^2; void lcd_init(); void cmd(unsigned char a); void dat(unsigned char b); void show(unsigned char *s); void delay(); void main() { int j; lcd_init(); show("embetronicx.com"); while(1) { cmd(0x18); for(j=0;j<25000;j++); } } void lcd_init() { cmd(0x02); cmd(0x28); cmd(0x0c); cmd(0x06); cmd(0x8f); } void cmd(unsigned char a) { rs=0; rw=0; P2&=0x0F; P2|=(a&0xf0); e=1; delay(); e=0; delay(); P2&=0x0f; P2|=(a<<4&0xf0); e=1; delay(); e=0; delay(); } void dat(unsigned char b) { rs=1; rw=0; P2&=0x0F; P2|=(b&0xf0); e=1; delay(); e=0; delay(); P2&=0x0f; P2|=(b<<4&0xf0); e=1; delay(); e=0; delay(); } void show(unsigned char *s) { while(*s) { dat(*s++); } } void delay() { unsigned int k,l; for(k=0;k<2;k++) for(l=0;l<1275;l++); }
OUTPUT
[ Please find the output image ]
Hope you learned something from this. In our next tutorial, we will see how to interface the DC motor with 8051.
You can also read the below tutorials.
|
|
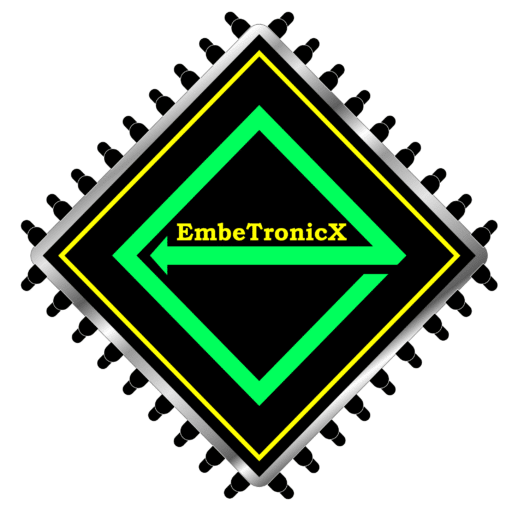
Embedded Software | Firmware | Linux Devic Deriver | RTOS
Hi, I am a tech blogger and an Embedded Engineer. I am always eager to learn and explore tech-related concepts. And also, I wanted to share my knowledge with everyone in a more straightforward way with easy practical examples. I strongly believe that learning by doing is more powerful than just learning by reading. I love to do experiments. If you want to help or support me on my journey, consider sharing my articles, or Buy me a Coffee! Thank you for reading my blog! Happy learning!