In our previous tutorial, we have interfaced 8051 with the SIM900a GSM module. In this tutorial, we are going to see RFID interfacing with 8051. So, We will use EM18 RFID Reader Module. Because it will give the data via serial communication. Before interfacing please have a look at How Does RFID Works?
Table of Contents
Suggestion to read
Why EM18?
EM-18 RFID reader is one of the commonly used RFID readers to read 125KHz tags. It features low cost, low power consumption, small form factor, and easy to use. It provides both UART and Wiegand26 output formats. It can be directly interfaced with microcontrollers using UART and with PC using an RS232 converter. Just power the module, and it will read any RFID card within range.
It will output the card’s ID in a serial string, which can easily be read by any microcontroller. The spacing on the pins is 2.54 mm, which means the module will directly fit on a breadboard.
Features
- 5V supply
- 125kHz read frequency
- EM4001 64-bit RFID tag compatible
- 9600bps TTL and RS232 output
- Magnetic stripe emulation output
- 100mm read range
RFID Interfacing With 8051
Circuit Diagram
The full circuit diagram for interfacing the RFID module to 8051 is shown below. The unique ID code in the RFID card is read by the circuit and displayed on the 16×2 LCD display.
LCD:
|
|
- RS – P0.5
- RW – P0.6
- EN – P0.7
- Data Lines – P2
EM18 (RFID Reader):
- RFID Reader TX – P3.0
Programming
Programming Steps
- Initialize the LCD
- Initialize the Serial Communication (9600 BaudRate)
- Receive the 12 bytes and display them into the LCD Display
In this tutorial, I’ve displayed the 12 bytes id. But you can play with that bytes. For example, You can verify the number. If it is matching you can run the motor.
Code
In this code, I’m reading the unique id number using the polling method. You can also try using a serial interrupt.
#include<reg51.h> #define lcd_data P2 sbit rs=P0^5; sbit rw=P0^6; sbit en=P0^7; void lcd_init(); void cmd(unsigned char a); void dat(unsigned char b); void show(unsigned char *s); void lcd_delay(); void ser_init(); void tx(unsigned char t); unsigned char rx(); void tx_str(unsigned char *s); unsigned char id[12]; unsigned int i; void main() { lcd_init(); ser_init(); cmd(0x80); show("<<SHOW UR CARD>>"); cmd(0xc0); for(i=0; i<12; i++) { id[i]=rx(); dat(id[i]); } while(1); } void lcd_init() { cmd(0x38); cmd(0x0e); cmd(0x01); cmd(0x06); cmd(0x0c); cmd(0x80); } void cmd(unsigned char a) { lcd_data=a; rs=0; rw=0; en=1; lcd_delay(); en=0; } void dat(unsigned char b) { lcd_data=b; rs=1; rw=0; en=1; lcd_delay(); en=0; } void show(unsigned char *s) { while(*s) { dat(*s++); } } void lcd_delay() { unsigned int lcd_delay; for(lcd_delay=0;lcd_delay<=6000;lcd_delay++); } void ser_init() { SCON=0x50; TMOD|=0x20; TH1=TL1=0xfd; TR1=1; } unsigned char rx() { while(RI==0); RI=0; return SBUF; } void tx(unsigned char t) { SBUF=t; while(TI==0); TI=0; } void tx_str(unsigned char *s) { while(*s) tx(*s++); }
You can try this code with hardware. In our next tutorial, we will interface the EEPROM with 8051 using the I2C communication.
|
|
You can also read the below tutorials.
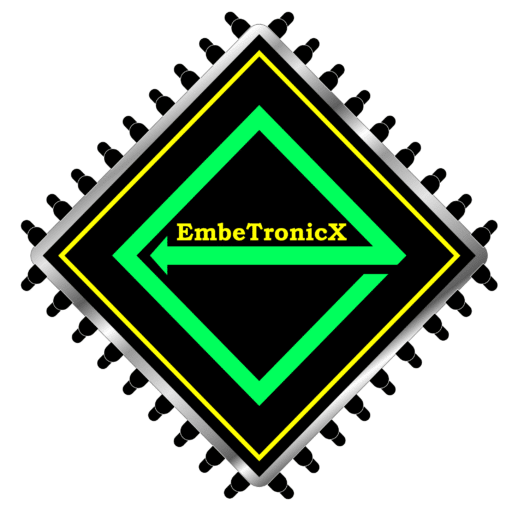
Embedded Software | Firmware | Linux Devic Deriver | RTOS
Hi, I am a tech blogger and an Embedded Engineer. I am always eager to learn and explore tech-related concepts. And also, I wanted to share my knowledge with everyone in a more straightforward way with easy practical examples. I strongly believe that learning by doing is more powerful than just learning by reading. I love to do experiments. If you want to help or support me on my journey, consider sharing my articles, or Buy me a Coffee! Thank you for reading my blog! Happy learning!