Hi All… In this tutorial, we are going to see the 8051 GPIO Tutorial. Before that, if you are not installing Keil please install that. If you don’t know please go through them here. Please see here if you don’t know how to create the project. See the introduction of 8051 Here.
Let’s start!!!!!
Before we start, please read this article to get to know how the GPIO is working in any microcontroller.
Table of Contents
8051 GPIO Tutorial
Introduction
Normally 8051 has four GPIO ports.
- Port 0 (P0)
- Port 1 (P1)
- Port 2 (P2)
- Port 3 (P3)
I have already discussed the Ports in the introduction. So now we can directly go into the programming.
|
|
WRITING ON A PORT PIN
Writing the data on a particular port is sending the data from the controller to any peripheral device which is connected with the controller. For example, here we are using the LED to indicate the data coming out from the parallel port.
READING FROM A PORT
Reading operation is getting the data from any peripheral device. Here we are using a simple push-button (switch) to give the input to the port pins.
LED Interfacing
A light-emitting diode (LED) is essentially a PN junction Opto-semiconductor that emits a monochromatic (single color) light when operated in a forward-biased direction. LEDs convert electrical energy into light energy. They are frequently used as “pilot” lights in electronic appliances to indicate whether the circuit is closed or not.
Pin Diagram
Here LEDs are connected to Port 2 (P2). If you are going to connect LEDs into Port 0 (P0) you should connect the pull-up resistor.
|
|
CODE
#include<reg51.h> void main() { unsigned int i; while(1) { P2=0x00; for(i=0;i<=30000;i++); P2=0xff; for(i=0;i<=30000;i++); } }
OUTPUT
Switch Interfacing
Pin Diagram
Here we are connecting the switch into Port 1 . 0 (P1.0). Then LEDs are connected to P2. Whenever I press the switch these LEDs should glow.
CODE
#include<reg51.h> sbit sw=P1^0; void main() { while(1) { if( sw == 0) { P2 = 0xFF; } else { P2 = 0x00; } } }
|
|
OUTPUT
[ Image Output ]
Hope you enjoyed this tutorial. But this is the basic. You can do whatever you want. I will give you some exercises. Do practice it.
Tasks
- Blink alternate LEDs at P3 using software delay
- Blink P0 LEDs in cyclic fashion using software delay
- Count the number of times a switch at P1.1 is pressed and display the count in P2If you have any doubt please comment below.
In our next tutorial, we will see LCD interfacing.
|
|
You can also read the below tutorials.
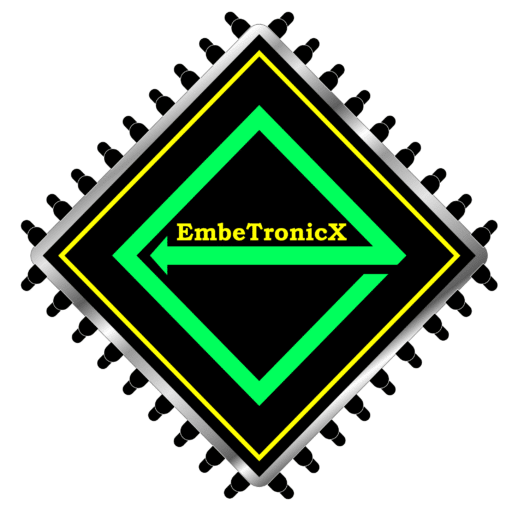
Embedded Software | Firmware | Linux Devic Deriver | RTOS
Hi, I am a tech blogger and an Embedded Engineer. I am always eager to learn and explore tech-related concepts. And also, I wanted to share my knowledge with everyone in a more straightforward way with easy practical examples. I strongly believe that learning by doing is more powerful than just learning by reading. I love to do experiments. If you want to help or support me on my journey, consider sharing my articles, or Buy me a Coffee! Thank you for reading my blog! Happy learning!