Hi Guys, In our previous tutorial, we have seen how to interface the Ultrasonic sensor with 8051. Today we will see GSM interfacing with 8051. Before that please take a look at GSM Module Introduction. And you should know the Serial Communication of 8051. I’ve posted that also. Please go through that (Serial Communication).
Table of Contents
GSM interfacing with 8051
SIM900A
GSM/GPRS Modem-RS232 is built with Dual Band GSM/GPRS engine- SIM900A works on frequencies 900/ 1800 MHz. The Modem is coming with an RS232 interface, which allows you to connect PC as well as a microcontroller with RS232 Chip(MAX232). The baud rate is configurable from 9600-115200 through AT command. The GSM/GPRS Modem is having an internal TCP/IP stack to enable you to connect with the internet via GPRS. It is suitable for SMS, Voice as well as DATA transfer applications in the M2M interface. You can read more about SIM900a.
Components Required
- GSM SIM900A module
- 8051 Development Board
Connection
Micro controller’s RX (P3.0) is connected to GSM module’s TX and Micro controller’s RX is Connected to GSM module’s RX pin.
Code
SMS Sending Code
This code is used to send the message to a particular number. You can also download this code from Github.
|
|
#include <reg51.h> #define NUMBER 0123456789 //Here insert your number where you want to send message void ser_init(); void tx(unsigned char send); void tx_str(unsigned char *s); unsigned char rx(); void sms(unsigned char *num1,unsigned char *msg); void gsm_delay(); unsigned int dell; int main() { ser_init(); sms(NUMBER, "Welcome to the Embetronicx"); while(1); } void ser_init() { SCON=0x50; TMOD=0x21; TH1=0xFD; TL1=0xFD; TR1=1; } void tx(unsigned char send) { SBUF=send; while(TI==0); TI=0; } void tx_str(unsigned char *s) { while(*s) tx(*s++); } unsigned char rx() { while(RI==0); RI=0; return SBUF; } void gsm_delay() { unsigned int gsm_del; for(gsm_del=0;gsm_del<=50000;gsm_del++); } void sms(unsigned char *num1,unsigned char *msg) { tx_str("AT"); tx(0x0d); gsm_delay(); tx_str("AT+CMGF=1"); tx(0x0d); gsm_delay(); tx_str("AT+CMGS="); tx('"'); while(*num1) tx(*num1++); tx('"'); tx(0x0d); gsm_delay(); while(*msg) tx(*msg++); tx(0x1a); gsm_delay(); }
Calling Code
This code is used to call a particular number. You can also download this code from Github.
#include <reg51.h> #define NUMBER 0123456789 //Here insert your number where you want to call void ser_init(); void tx(unsigned char send); void tx_str(unsigned char *s); unsigned char rx(); void sms(unsigned char *num1,unsigned char *msg); void gsm_delay(); unsigned int dell; int main() { ser_init(); call(NUMBER); while(1); } void ser_init() { SCON=0x50; TMOD=0x21; TH1=0xFD; TL1=0xFD; TR1=1; } void tx(unsigned char send) { SBUF=send; while(TI==0); TI=0; } void tx_str(unsigned char *s) { while(*s) tx(*s++); } unsigned char rx() { while(RI==0); RI=0; return SBUF; } void gsm_delay() { unsigned int gsm_del; for(gsm_del=0;gsm_del<=50000;gsm_del++); } void call(unsigned char *num2) { tx_str("AT"); tx(0x0d); gsm_delay(); tx_str("AT+CMGF=1"); tx(0x0d); gsm_delay(); tx_str("ATD"); while(*num2) tx(*num2++); tx(';'); tx(0x0d); for(dell=0;dell<=30;dell++) gsm_delay(); tx_str("ATH"); tx(0x0d); gsm_delay(); }
In our next tutorial, we will see how to interface the RFID with 8051.
You can also read the below tutorials.
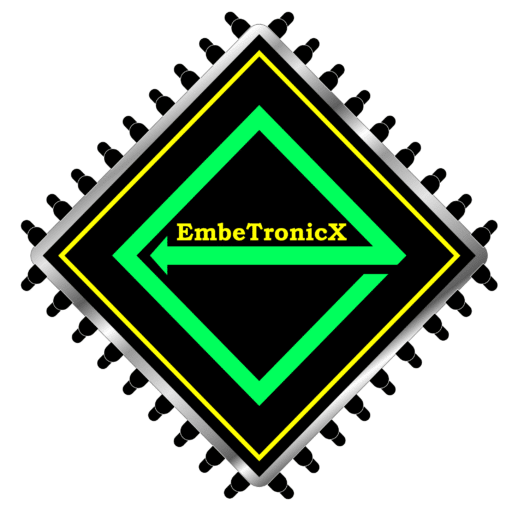
Embedded Software | Firmware | Linux Devic Deriver | RTOS
Hi, I am a tech blogger and an Embedded Engineer. I am always eager to learn and explore tech-related concepts. And also, I wanted to share my knowledge with everyone in a more straightforward way with easy practical examples. I strongly believe that learning by doing is more powerful than just learning by reading. I love to do experiments. If you want to help or support me on my journey, consider sharing my articles, or Buy me a Coffee! Thank you for reading my blog! Happy learning!