This article is a Series on the C programming tutorial and carries the discussion on C language programming and its implementation. In our previous article, we discussed Jump statements in the C program like break, continue, goto. In this article, we are going to explain Arrays in C. It aims to provide easy and practical examples for understanding the C program.
Table of Contents
What is an array?
An array is a linear data structure, and it is used to store the collection of similar data in a contiguous memory location. The array can hold only similar data types. For example, if you create an integer array, you can store multiple integers not the other data types like char, float, etc.
Arrays provide a way to efficiently organize and access a large amount of data. Each element in an array is identified by its index, which represents its position in the array. Arrays are widely used in programming for tasks such as storing and manipulating data, implementing algorithms, and working with collections of objects.
What are the types of Array in C?
There are three types of arrays:
We will see these types one by one.
|
|
One Dimensional Array (1D Array) in C
What is a One-Dimensional Array?
It is the simplest form of an array. It is a linear collection of elements of the same data type. The elements are stored in contiguous memory locations and accessed by a single index. It is represented by a single subscript enclosed in square brackets [ ], e.g., int arr[5];.
Syntax
The syntax of a one-dimensional array in C is as follows:
datatype array_name[size];
Where:
datatype
is the type of data that the array will hold, such as int, float, char, etc.array_name
is the name of the array.size
is the number of elements that the array can hold.
Array Declaration
For example, the syntax for declaring an integer array of size 5 would be:
int arr[5];
This declares an array named arr
that can hold 5 integer values. If we don’t initialize the elements, all the elements will have garbage values.
|
|
If you want to add the values at the initialization time, then the declaration would be,
int arr[5] = {10,20,30,40,50};
Another way of declaration is Partial declaration or initialization. The partial declaration would be,
int arr[5] = {10,20,30};
In the above declaration, we have initialized the first three elements. What will happen to the other elements? The rest of the element will be initialized as 0.
We can also initialize the array variable without setting the size of an array. The compiler will automatically calculate the size of an array. But initialization values must be there in this case.
int student[] = {89, 76, 98, 91, 84};
Here are some examples of valid array declarations.
|
|
Example
int arr[5];
int arr[5] = {1, 2, 3, 4, 5};
int arr[5] = {1, 2};
char arr[5] = {'a', 'b', 'c', 'd', 'e'};
float arr[3] = {1.0, 2.5, 3.9};
int arr[] = {89, 76, 98, 91, 84};
Invalid Array declaration
Here are some examples of invalid array declarations.
Example
int arr[];
int arr[5] = {'1', '2', '3', '4', '5'};
int arr[5] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
char arr[5] = {a, b, c, d, e};
char arr[2] = {"Invalid", "array"};
How to assign a value to the array?
To assign a value to an array in C, you can use either of the following methods:
- Assigning values at the time of declaration:
int arr[5] = {10, 20, 30, 40, 50};
This assigns the values 10, 20, 30, 40, and 50 to the elements of the
arr
array. - Assigning values one by one using the assignment operator:
int arr[5]; arr[0] = 10; arr[1] = 20; arr[2] = 30; arr[3] = 40; arr[4] = 50;
This assigns values individually to each element of the
arr
array by specifying the index of the element starting from 0.
Remember to replace int
with the appropriate data type if you are using an array of a different data type, such as char
, float
, etc.
How to access an array?
To access an element in an array, you use the array name followed by square brackets [] containing the index of the element you want to access. The index represents the position of the element in the array, starting from 0 for the first element.
Here is an example of how to access elements in an array:
int arr[5] = {10, 20, 30, 40, 50}; // Accessing elements of the array int firstElement = arr[0]; // Accessing the first element int thirdElement = arr[2]; // Accessing the third element printf("First element of the array: %d\n", firstElement); printf("Third element of the array: %d\n", thirdElement);
Output:
|
|
First element of the array: 10 Third element of the array: 30
In this example, the array arr
is composed of 5 elements. Using the square brackets and the index number, we can access specific elements in the array. The value of the first element (at index 0) is stored in the variable firstElement
, and the value of the third element (at index 2) is stored in the variable thirdElement
.
How the memories are allocated for an array?
Assume that I want to store five integer values. One way is creating five integer variables (int a,b,c,d,e;)
. Another way is creating an integer array with 5 elements (int arr[5];
). If the integer integer occupies 4 bytes in memory, then in both ways it will allocate 20 bytes. Then what is the difference?
If we use integer variables, then the memory location will not necessarily be contiguous memory. That means the variable ‘a’ will be in one location and the variable 'b'
will be in another location etc. But in arrays, it will be contiguous 20 bytes. Another difference is while accessing each value, we have to use the variable name (a,b,c,d,e
). But in the array, we can access something like arr[0]
, arr[1]
, arr[2]
, etc
Memory allocation for an array depends on the data type and size of the array. The memory is allocated in a contiguous block, which means the elements of the array are stored in consecutive memory locations.
Let’s consider an example of an integer array with a size of 5:
|
|
int arr[5];
Assuming that an integer occupies 4 bytes in memory, the memory allocation for this array would be:
Memory Address Value 1000 Uninitialized 1004 Uninitialized 1008 Uninitialized 1012 Uninitialized 1016 Uninitialized
If we initialize the array at the time of declaration, for example:
int arr[5] = {10, 20, 30, 40, 50};
Then the memory allocation and initialization would be:
Memory Address Value 1000 10 1004 20 1008 30 1012 40 1016 50
So, the memory allocation for an array is done in a sequential manner, and each element of the array is stored at its respective memory location based on the size of the data type.
Array Manipulation
You can perform various operations on one-dimensional arrays, such as:
|
|
- Assigning values to array elements
- Accessing array elements
- Modifying array elements
- Searching for a specific value
- Sorting the array
- Performing calculations on array elements
- Printing the array elements
One-dimensional arrays are a fundamental data structure in C programming and are widely used for storing and manipulating collections of data efficiently. They provide a convenient and organized way to access and manage multiple values of the same data type.
Example Program
Here’s a program that demonstrates various operations on one-dimensional arrays in C:
#include <stdio.h> // Function to print the elements of an array void printArray(int arr[], int size) { for (int i = 0; i < size; i++) { printf("%d ", arr[i]); } printf("\n"); } int main() { int arr[5] = {5, 2, 8, 1, 6}; // Declare and initialize the array // Printing the initial array printf("Initial array: "); printArray(arr, 5); // Modifying an element at a specific index arr[3] = 9; printf("Array after modifying element at index 3: "); printArray(arr, 5); // Accessing an element at a specific index int element = arr[2]; printf("Element at index 2: %d\n", element); // Finding the size of the array int size = sizeof(arr) / sizeof(arr[0]); printf("Size of the array: %d\n", size); // Searching for a specific value in the array int target = 8; int found = 0; for (int i = 0; i < size; i++) { if (arr[i] == target) { found = 1; break; } } if (found) { printf("The value %d is present in the array.\n", target); } else { printf("The value %d is not present in the array.\n", target); } // Sorting the array in ascending order using bubble sort for (int i = 0; i < size - 1; i++) { for (int j = 0; j < size - i - 1; j++) { if (arr[j] > arr[j + 1]) { // Swap elements int temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } } printf("Array after sorting in ascending order: "); printArray(arr, 5); // Performing calculations on array elements int sum = 0; for (int i = 0; i < size; i++) { sum += arr[i]; } printf("Sum of all elements: %d\n", sum); // Printing the array in reverse order printf("Array in reverse order: "); for (int i = size - 1; i >= 0; i--) { printf("%d ", arr[i]); } printf("\n"); return 0; }
This program demonstrates various array manipulations such as modifying elements, accessing elements, finding the size of the array, searching for a specific value, sorting the array, performing calculations on array elements, and printing the array in reverse order.
Output:
Initial array: 5 2 8 1 6 Array after modifying element at index 3: 5 2 8 9 6 Element at index 2: 8 Size of the array: 5 The value 8 is present in the array. Array after sorting in ascending order: 2 5 6 8 9 Sum of all elements: 30 Array in reverse order: 9 8 6 5 2
Two-Dimensional Array (2D Array) in C
What is a Two-Dimensional Array?
A two-dimensional array is an array of arrays in which each element can be accessed using two indices, representing rows and columns. It is used to represent tabular data or a matrix-like structure with rows and columns.
|
|
In a two-dimensional array, the elements are stored in a contiguous block of memory, where each row is stored in one array and rows are stored sequentially. This allows efficient access to individual elements using the row and column indices.
Syntax
The syntax for declaring a two-dimensional array in C is:
datatype array_name[row_size][column_size];
Here, the datatype
specifies the type of data that the array will hold, such as int
, float
, char
, etc. The array_name
is the name of the array, and row_size
and column_size
specify the number of rows and columns in the array, respectively.
Initialization of Two-Dimensional Array in C
You can initialize a two-dimensional array during declaration by providing the initial values in curly braces {}
. For example:
int matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
This creates a two-dimensional array named matrix
with 3 rows and 3 columns and initializes the elements with the given values. We can use the below way also to initialize the two-dimensional array.
|
|
int matrix[3][3] = {1, 2, 3, 4, 5, 6, 7, 8, 9};
To access an element in a two-dimensional array, you use the row and column indices. For example, to access the element at row i
and column j
, you can use array_name[i][j]
. The indices are zero-based, so the first row and column have indices 0.
Two-dimensional arrays are commonly used in various applications, such as representing grids, matrices, and tables of data. They provide a convenient way to organize and manipulate data in a tabular structure.
Two-Dimensional Arrays Program
#include <stdio.h> int main() { int i, j, matrix[3][3] = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}; for (i = 0; i < 3; i++) { for(j = 0; j < 3; j++) { printf("%2d ", matrix[i] [j]); } printf("\n"); } return 0; }
1 2 3 4 5 6 7 8 9
How the data is stored in the two-dimensional array in C?
A two-dimensional array is stored in memory as a contiguous block of data. The elements of the array are organized in rows and columns. The memory is allocated in a sequential manner to ensure efficient data access.
To understand how the data is stored in a two-dimensional array, consider the following example:
|
|
int matrix[3][3] = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
Let’s assume that an integer occupies 4 bytes in memory. The data looks like below.
C0 | C1 | C2 | |
R0 | 1 | 2 | 3 |
R1 | 4 | 5 | 6 |
R2 | 7 | 8 | 9 |
The memory allocation for this two-dimensional array would be as follows:
Memory Address Value 1000 1 1004 2 1008 3 1012 4 1016 5 1020 6 1024 7 1028 8 1032 9
In this example, the matrix
array has 3 rows and 3 columns. Each element is an integer, so each element occupies 4 bytes in memory. The memory addresses are assigned in ascending order, and the elements are stored one after another in a sequential manner.
When accessing an element in the two-dimensional array, the row and column indices are used to calculate the memory address of the desired element. For example, to access the element at row 1 and column 2 (value 2), the memory address would be matrix[1][2]
or 1012
.
By storing the elements in a contiguous block of memory, accessing elements in a two-dimensional array can be done efficiently using simple calculations based on the indices. This memory layout enhances data retrieval speed and reduces memory fragmentation.
|
|
Multi-Dimensional Array in C
A multi-dimensional array is a data structure that can store elements in multiple dimensions or layers. Unlike a one-dimensional array, which has a linear structure, a multi-dimensional array organizes data in the form of a matrix or table. Technically, a two-dimensional array is also a multi-dimensional array. But we have discussed it earlier. So, we will see a 3D array.
A 3D array in C is a multidimensional array that has three dimensions: rows, columns, and depth. It is used to store and manipulate data that requires three levels of indexing or representation.
Just as a 2D array represents a grid or a matrix, a 3D array can be thought of as a collection of multiple grids or matrices stacked on top of each other along the depth dimension.
The syntax for declaring a 3D array in C is as follows:
datatype array_name[size1][size2][size3];
Here, datatype
represents the type of data that the array will hold, such as int
, float
, char
, etc. array_name
is the name of the array, and size1
, size2
, and size3
represent the number of elements in each dimension.
|
|
For example, to declare a 3D array of integers with dimensions 2x3x4, you would write:
int array[2][3][4];
This creates a 3D array named array
with 2 rows, 3 columns, and 4 depth levels.
To access an element in a 3D array, you need to specify the indices for each dimension. For example, to access the element at row 1, column 2, and depth 3, you would write:
int element = array[1][2][3];
You can perform various operations on a 3D array, such as initializing its elements, modifying the values of specific elements, and traversing through the array using nested loops. This allows you to manipulate and analyze 3D data structures, such as cubes, volumes, and 3D grids.
How the data is stored in the three-dimensional array in C?
In a three-dimensional (3D) array in C, data is stored in a contiguous block of memory, similar to a two-dimensional array. However, the organization of elements in a 3D array is more complex, as it involves three dimensions: rows, columns, and depth.
|
|
To understand how the data is stored in a 3D array, consider the following example:
int array[2][3][4] = { {{1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12}}, {{13, 14, 15, 16}, {17, 18, 19, 20}, {21, 22, 23, 24}} };
Let’s assume that an integer occupies 4 bytes in memory. The memory layout for this 3D array would be represented as follows:
Array[0][0] Array[0][1] Array[0][2] Array[1][0] Array[1][1] Array[1][2] | | | | | | V V V V V V Memory Address Value Memory Address Value Memory Address Value Memory Address Value Memory Address Value Memory Address Value ------------------------------------------------------------------------------------------- 1000 1 1004 2 1008 3 1012 4 1016 5 1020 6 1024 7 1028 8 1032 9 1036 10 1040 11 1044 12 1048 13 1052 14 1056 15 1060 16 1064 17 1068 18 1072 19 1076 20 1080 21 1084 22 1088 23 1092 24
In this example, the array
3D array has 2 rows, 3 columns, and 4 depth levels. Each element is an integer, so each element occupies 4 bytes in memory. The memory addresses are assigned in ascending order, and the elements are stored one after another in a sequential manner.
When accessing an element in the 3D array, you need to specify the indices for each dimension. For example, to access the element at row 0, column 1, and depth 2 (value 7), you would write:
int element = array[0][1][2];
By storing the elements in a contiguous block of memory, accessing elements in a 3D array can be done efficiently using simple calculations based on the indices. This memory layout enhances data retrieval speed and reduces memory fragmentation.
|
|
It’s important to note that working with higher-dimensional arrays can increase the complexity of your code and may require more memory. It’s crucial to carefully consider the size and usage requirements of your array to ensure efficient memory utilization and avoid potential memory overflows.
Overall, 3D arrays in C provide a powerful tool for representing and manipulating 3D data structures, enabling you to work with multidimensional data in a systematic and organized manner.
Advantages of Array in C
Arrays in C offer several advantages:
- Efficient Data Storage: Arrays allow you to store multiple values of the same data type in a contiguous block of memory, resulting in efficient memory utilization. This is particularly useful when dealing with large amounts of data.
- Random Access: Arrays provide direct access to individual elements using their index. This allows for efficient retrieval, modification, and manipulation of array elements, without the need to iterate through the entire array.
- Simplified Algorithms: Many algorithms, such as sorting and searching, are simplified when implemented with arrays. Array operations can be optimized through various techniques like binary search, which drastically reduces the time complexity of searching for a specific element.
- Efficient Iteration: With arrays, you can easily iterate over the elements using loops like
for
orwhile
, making it convenient to perform repetitive operations on every element in the array. This reduces the need for redundant code and makes the program more efficient. - Flexibility: Arrays provide flexibility in data organization and manipulation. They allow you to create multidimensional arrays, which are useful for representing complex data structures like matrices or grids. Arrays can also be resized dynamically using pointers and memory allocation functions, providing flexibility in managing data.
- Easy to Implement Data Structures: Arrays form the basis for implementing various data structures like stacks, queues, and linked lists. Data structures built with arrays enable efficient storage, retrieval, and manipulation of data in specific patterns, enhancing program efficiency and organization.
- Fast and Efficient: Arrays offer fast access to elements, as their memory locations are contiguous. This enables efficient memory caching and improves data retrieval speed compared to other data structures that might require traversing pointers or linked nodes.
- Memory Efficiency: When compared to dynamically allocated data structures, arrays are more memory-efficient. They require less memory overhead because the memory allocation is straightforward and there are no additional data structures, such as linked lists or trees, to manage.
- Ease of Implementation: Arrays are simple to understand and implement in C. They require minimal programming knowledge and can be easily implemented even by beginners. This simplicity makes arrays a popular choice for various programming tasks.
- Compatible with Library Functions: Arrays are widely used in C and are compatible with many library functions and algorithms. Various libraries and APIs provide extensive support for array manipulation, making it easier to perform complex operations on array elements.
Overall, arrays are a versatile data structure in C, providing efficient storage, fast access to elements, simplicity in implementation, and compatibility with algorithms and data structures. They are an essential tool for managing and manipulating data in various programming scenarios.
Applications of an Array in C
Arrays are a fundamental data structure in C programming and have various applications. Some common applications of arrays in C include:
|
|
- Storing and Manipulating Data: Arrays provide a convenient way to store and manipulate a collection of data of the same type. For example, you can use an array to store the scores of students in a class, the temperatures recorded over a period of time, or the coordinates of points in a graph.
- Implementing Algorithms: Arrays are often used to implement various algorithms. Sorting algorithms like Bubble Sort, Insertion Sort, and Selection Sort can be easily implemented using arrays. Additionally, searching algorithms like Linear Search and Binary Search can be efficiently implemented using arrays.
- Working with Matrices and Grids: In scientific and mathematical computations, arrays are widely used to represent matrices and grids. Matrices can be used to perform operations such as matrix multiplication, addition, and subtraction. Grids can be used to represent game boards, visualize data, or solve optimization problems.
- Managing and Analyzing Data Structures: Arrays are used to build more complex data structures, such as stacks, queues, heaps, and hash tables. These data structures are essential for managing and analyzing data in various applications, including computer science, databases, and networking.
- Image Processing and Signal Processing: Arrays are heavily used in image processing and signal processing applications. Images and signals are represented as two-dimensional or one-dimensional arrays, and various operations, such as filtering, enhancement, and compression, are applied to the elements of the arrays.
- Working with Strings: Strings in C are represented as arrays of characters. Arrays make it easy to manipulate strings, such as concatenation, searching for substrings, and conversion between different string formats. Arrays also allow for efficient storage and retrieval of individual characters in strings.
- Implementing Data Structures: Arrays can be used to implement fundamental data structures like stacks, queues, linked lists, and trees. These data structures are widely used for organizing and managing data in various applications.
- Numerical Computations: Arrays are essential for numerical computations, as they enable efficient manipulation of large sets of numerical data. Numerical operations like summation, averaging, and statistical analysis can be performed on elements of an array.
These are just a few examples of the many applications of arrays in C programming. Arrays provide a versatile and efficient way to store, manipulate, and access data, and they form the backbone of many algorithms and data structures used in computer programming.
You can also read Flexible Array Member in C programming.
In our next tutorial, we will discuss about strings in C programming.
Please read the below tutorials.
|
|
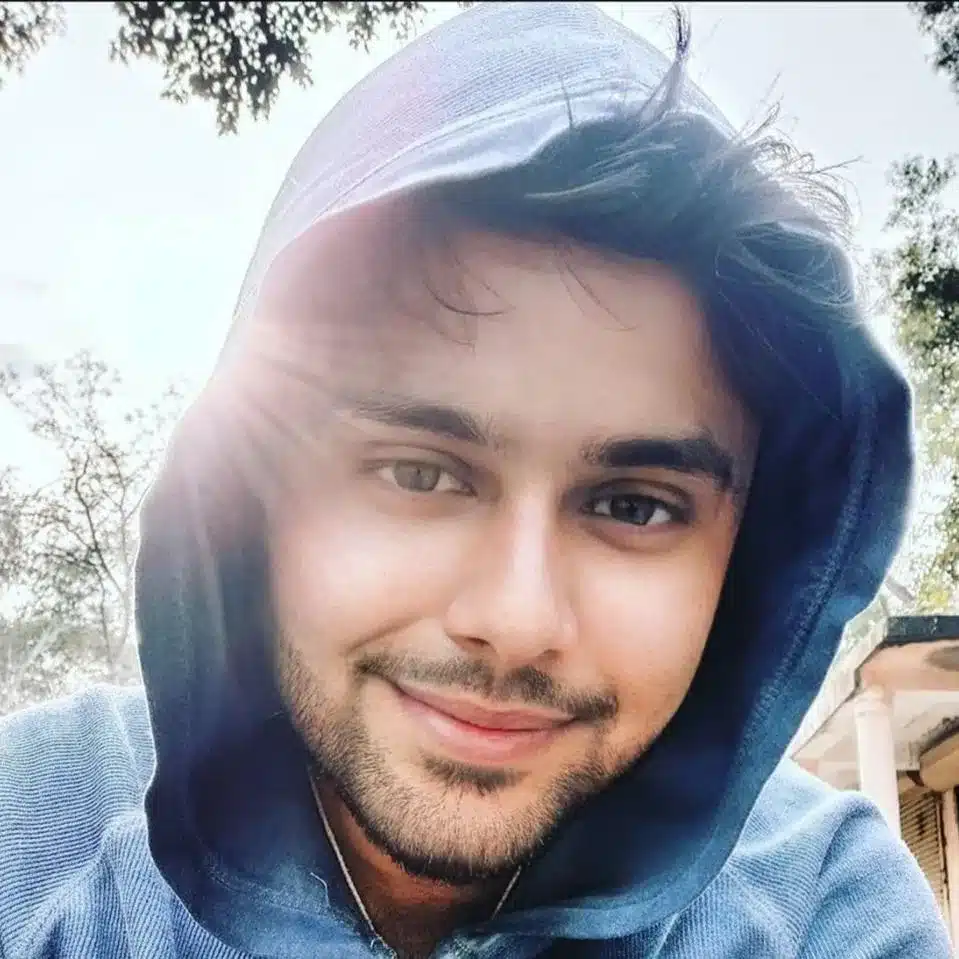
Embedded Engineer|| C programming || Microcontroller
Hi. I am Sunil an Electrical Engineer as well as an Embedded Engineer. I had interested in embedded systems to learn things and to implement my working ideas practically on embedded and all their domains. So here you can find my articles which have been created by me in a simple way to make you understand its concepts. I believe that learning things and after that implementing them practically is a good way to gain experience.
Thank you for reading my articles keep supporting and keep learning!