This article is the continuation of the Series on the C programming tutorial and carries the discussion on C language programming and its implementation. It aims to provide easy and practical examples for understanding the C program. In our last article, we have seen the Storage class in C programming. In this article, we are going to explain Functions in C Programming.
Table of Contents
What are the functions in C Programming?
They can be classified into library functions, user-defined functions, and built-in functions. Library functions are predefined functions provided by C libraries, while user-defined functions are created by the programmer. Built-in functions are specific to the C language and perform various operations, such as input/output, string manipulation, memory allocation, and mathematical computations.
A function is a block of code that performs a specific task enclosed within curly brackets {}
that takes inputs, does the processing of code, and provides the resultant output. or Functions are small module of the program which is used to reduce the size of the main program in C programming language.
C programming language provides a variety of functions that help perform specific tasks. Functions in C programming enable code reusability, and modular programming, and improve code readability. They encapsulate a series of instructions into a single unit that can be called whenever required. Functions in C are declared using a return type, function name, and optional parameters.
What are the uses of the functions in C programming?
The functions in C programming are used for various purposes such as,
|
|
- It is used to avoid rewriting the same code again and again in the program (code reusability).
- It can be called many numbers of times from any place of program blocks.
- It makes a code modular.
- It becomes easy to understand and manage.
- It improves the code readability and organization of the code.
- They allow programmers to break down complex tasks into smaller, manageable parts, making it easier to understand and maintain the code.
- Functions in C can be used to perform calculations, process data, manipulate strings, perform input/output operations, and much more.
Syntax
return_type fun_name( data_type parameter1, data_type parameter2, ... ); { //Statements //return value should match the return type of the function //if the function return type is void, then we can't return any value return (value); }
Before jumping to the functions in C, we will have to know a few things,
- Return type
- Function name
- Parameters
- Return value
- Function prototype
- Function definition
- Function calling
Return type
The return type of a function in the C programming language determines the type of data that the function will return when it is executed. So, if the function return type is int
, then the function return value can be only integers. We can’t return float or other data types. If the function return type is void
, then the function returns no value or expression.
Function Name
In C programming, a function name is a unique identifier that is used to refer to a specific block of code that performs a specific task. It is important to choose descriptive and meaningful names for functions to enhance code readability and maintainability.
Parameters
Parameters in C functions are values that are passed into the function when it is called. They allow the function to perform specific operations based on the values provided. Parameters are specified within the parentheses of a function declaration and can have different data types, such as int, float, char, etc. When the function is called, the actual values passed into the parameters are called arguments. The function can then use these arguments to perform its intended tasks.
Types of Parameters
Parameters are divided into two types,
|
|
Actual Parameters
Actual parameters in C refer to the values that are passed to a function when it is called. These values are provided by the caller of the function and are used by the function to perform its tasks. The actual parameters are passed to the function through its arguments, which are specified in the function’s declaration. By using actual parameters, programmers can pass information between different parts of their program and perform various operations. Overall, actual parameters play a crucial role in C programming by enabling the passing of data to functions.
Formal Parameters
Formal parameters in C refer to the variables that are declared in the function definition. They receive values from the actual parameters or arguments that are passed when the function is called. The formal parameters act as placeholders for these values within the function body. They allow data to be passed into functions and enable the function to perform operations on that data. The scope of formal parameters is limited to the function where they are declared, ensuring encapsulation and preventing conflicts with variables outside the function.
Example
#include <stdio.h> int add(int n1,int n2); int main() { int a = 10; int b = b; int sum = add(a,b); // <------- (a, b) Actual Paramters printf("%d + %d = %d",a,b,sum); return 0; } int add(int n1,int n2) { return (n1 + n2); // <------- (n1, n2) Formal Paramters }
We can pass the value in function two ways call by value or call by reference. We can see these topics later in this article.
Return value
In the C programming language, the return value refers to the value that a function sends back to the caller after it has finished executing. It is specified in the function’s declaration and can be of any valid data type in C. When a function returns a value, it can be assigned to a variable or used directly in expressions within the calling code. The return value is a way for functions to provide the result or outcome of their execution to the rest of the program. We have already discussed this return keyword in this article.
|
|
Function Prototype in C
A function prototype in C is a declaration of a function that describes its name, return type, and parameters. It serves as a blueprint for the function and helps the compiler to verify the function calls and their usage in the program. This is also called as the function declaration. While writing parameter names during declaration is optional. So, just the data type is enough.
This function prototype doesn’t require any statements. Because memory won’t be allocated this time. This prototype helps the compiler to identify that the function is defined somewhere else. So, the compiler doesn’t throw a warning or error.
Syntax
return_type name_of_function(datatype_parameter_1, datatype_parameter_2);
Example
int add(int n1,int n2); or int add(int,int);
Function Definition in C
A function definition in the C programming language is a block of code that specifies the actions or operations to be executed when the function is called. It includes the name of the function, the input parameters (if any), the return type (if any), and the actual code that performs the desired actions. Function definitions allow programmers to break down their code into modular and reusable components, making it easier to organize and maintain large projects.
|
|
Example
int add(int n1,int n2) // Function Definition { return (n1 + n2); }
Function calling in C
Function calling in C refers to the process of invoking or executing a function within a C program. When a function is called, the program transfers control to that function, which performs a specific task or computation. The calling function passes arguments or parameters to the called function, if required. After the called function finishes its execution, the control returns back to the calling function, allowing it to continue from where it left off. A function call is a fundamental concept in C programming and is used to organize code into modular and reusable components.
Syntax
function_name(parameters);
Example
int sum = add(a,b);
Example
#include <stdio.h> int add(int n1,int n2); //Function Declaration int main() { int a,b,sum; printf("Enter two number:- "); scanf("%d%d",&a,&b); sum = add(a,b); //Function Calling printf("%d + %d = %d",a,b,sum); return 0; } int add(int n1,int n2) // Function Definition { return (n1 + n2); }
Output
|
|
Enter two number:- 10 5 10 + 5 = 15
What are the types of functions?
There are two types of functions available in C programming.
Pre-defined Function
A predefined function in C refers to a function that is already defined in the C programming language and can be used directly without needing to be declared or implemented again. These functions are built-in and provide commonly used functionalities such as mathematical calculations, string manipulations, input/output operations, and memory management. They are included in standard C libraries and can be called by their respective function names. Predefined functions help in simplifying the coding process and enable programmers to accomplish various tasks efficiently. C has around 32,000 inbuilt functions stored. The pre-defined function is also known as In-built/derived/standard library function. You can easily call them without defining them as they are already defined.
Example
strcmp(),
strcpy(),
printf(),
scanf() etc
.
User-defined Function
A user-defined function in C is a function that is created by the user rather than being built-in into the programming language. It allows the user to define their own functions with specific functionality, which can be called and executed within the C program. User-defined functions provide the ability to write modular and reusable code, enhancing the overall structure and organization of the program. It increases the scope and functionality, and reusability it can define and use any function when a user wants.
Example
#include <stdio.h> int sum(int a, int b); int main() { int a, b, res; printf("Enter two number:- "); scanf("%d%d",&a,&b); res = sum(a, b); printf("%d + %d = %d",a,b,res); return 0; } int sum(int a, int b) { return a + b; }
Output
|
|
Enter two number:- 10 20 10 + 20 = 30
Combinations of function creation
We can create the functions as per our wish. But mostly we will end up with the below combinations.
- Function without return value and without arguments
- Functions with return value and without arguments
- Functions without return value and with arguments
- Functions with return value and arguments
Function without return values and without arguments
In this example, the function doesn’t have any return values and arguments.
Example
#include <stdio.h> void test_fn( void ); int main() { test_fn(); return 0; } void test_fn( void ) { printf("This is Test Function\n"); }
Functions with return value and without arguments
In this example, the function doesn’t have arguments and it has a return value.
#include <stdio.h> int test_fn( void ); int main() { int ret = test_fn(); return 0; } int test_fn( void ) { printf("This is Test Function\n"); return 0; }
Functions without return value and with arguments
In this example, the function doesn’t have a return value and it has an argument(s).
|
|
#include <stdio.h> void test_fn( int arg1, int arg2 ); int main() { test_fn( 10, 12 ); return 0; } void test_fn( int arg1, int arg2 ) { printf("This is Test Function - %d %d\n", arg1, arg2); }
Functions with return value and arguments
In this example, the function has a return value, and an argument(s).
#include <stdio.h> int test_fn( int arg1, int arg2 ); int main() { int ret = test_fn( 10, 12 ); return 0; } int test_fn( int arg1, int arg2 ) { printf("This is Test Function - %d %d\n", arg1, arg2); return 0; }
What is Recursive Function in C?
A recursive function in C is a function that calls itself during its execution. This can be useful for solving problems that can be broken down into smaller, similar subproblems. By designing a recursive function, you can tackle complex tasks in a simpler and more organized manner. The function will continue calling itself until a base case is reached, at which point the recursion will stop.
Example
#include <stdio.h> int fact(int n); int main() { int n; printf("Enter a Number:- "); scanf("%d",&n); printf("Factorial of %d is %d",n,fact(n)); } int fact(int n) { if(n<=1) { return 1; } else { n=n*fact(n-1); } return (n); }
Output
Enter a Number:- 5 Factorial of 5 is 120
In the above function, we are calling the fact()
function within that function. So, that will be called continuously until the n
is equal to 1.
|
|
Is the recursion function safe to use in C programming?
The use of recursion in C programming can be considered safe as long as it is implemented correctly. Recursion is a technique where a function calls itself repeatedly until a base case is reached. It can be a powerful tool for solving complex problems, but it should be used with caution to avoid potential issues such as stack overflow or infinite loops. Proper termination conditions and careful management of memory are essential when using recursive functions in C programming.
What are the problems of recursive functions in C?
Recursive functions in C can be a powerful tool for solving certain problems, but they can also present some challenges. Here are a few potential problems you may encounter when using recursive functions in C:
- Stack Overflow: Recursive functions rely on the call stack to keep track of function calls. If the recursion depth becomes too large or if the function doesn’t have a proper termination condition, it can lead to a stack overflow. This can cause your program to crash.
- Performance Overhead: Recursive functions can have higher performance overhead compared to iterative solutions. Each recursive call requires additional memory and function call overhead. For large inputs, this can significantly impact performance.
- Limited Stack Size: The size of the call stack in C is finite. If your program requires a large number of recursive calls or if each recursive call consumes a significant amount of stack space, you may run into stack size limitations. This can result in a stack overflow error.
- Code Complexity: Recursive functions can be more complex to understand and debug compared to iterative solutions. It’s important to have a clear understanding of how the recursion unfolds and ensure that the termination conditions are properly defined.
- Potential Infinite Recursion: If the termination condition is not correctly defined or if there is a logical error in the implementation, it’s possible to end up with infinite recursion. This means the function will keep calling itself indefinitely, leading to an infinite loop.
To use recursive functions effectively, it’s important to understand these potential issues and to design your functions with appropriate termination conditions and input validation checks.
Call by Value
Call by value is a method of passing arguments to a function in programming languages, including C. In this method, the value of the argument is copied and passed to the function. This means that any changes made to the argument within the function do not affect the original value outside of the function.
Actual arguments and formal arguments are created in separate memory locations in this method.
|
|
Example
#include <stdio.h> int test_fn( int arg1, int arg2 ); int main() { int a = 10, b = 12; printf("This is Main Function before function call - %d, %d\n", a, b); int ret = test_fn( a, b); printf("This is Main Function after function call - %d, %d\n", a, b); return 0; } int test_fn( int arg1, int arg2 ) { arg1 = 20; arg2 = 30; printf("This is Test Function - %d %d\n", arg1, arg2); return 0; }
If you see the above program, we are passing the variable a, b
to the function called test_fn
. In the test_fn
, we are changing the value of arg1, arg2
(Formal parameters which receive the value from a
and b
). But after the test_fn
returns, we are printing the value of a, b
. But it is still having 10 and 12 respectively. So, the change that we did inside the test_fn
doesn’t affect the actual parameters. This method is called Call by value.
Output
This is Main Function before function call - 10, 12 This is Test Function - 20 30 This is Main Function after function call - 10, 12
Call by Reference
Call by reference is a method that allows a function to modify the actual value of a variable by passing its memory address instead of the value itself. This means that any changes made to the variable within the function will also affect the original variable outside the function. This is done using the pointer. We have discussed the pointer completely in this article.
Actual arguments and formal arguments share the same memory locations and we are passing the address of the memory location to the function.
Example
#include <stdio.h> int test_fn( int *arg1, int *arg2 ); int main() { int a = 10, b = 12; printf("This is Main Function before function call - %d, %d\n", a, b); int ret = test_fn( &a, &b); printf("This is Main Function after function call - %d, %d\n", a, b); return 0; } int test_fn( int *arg1, int *arg2 ) { *arg1 = 20; *arg2 = 30; printf("This is Test Function - %d %d\n", *arg1, *arg2); return 0; }
If you see the above program, we are passing the address of a, b
to the function called test_fn
. In the test_fn
, we are changing the value of arg1, arg2
using the pointer. As we are changing the value in the memory itself (using a pointer), The actual parameters value also will be changed. This method is called Call by reference.
|
|
Output
This is Main Function before function call - 10, 12 This is Test Function - 20 30 This is Main Function after function call - 20, 30
Frequently Asked Question
What is a function in C?
A function is a block of code that performs a specific task enclosed within curly brackets {}
that takes inputs, does the processing of code, and provides the resultant output.
What are the actual parameter and formal parameters?
In programming, when a function or method is called, actual parameters are the values or variables that are passed as arguments to the function. These values are specific to the particular function call and may be different each time the function is called. On the other hand, formal parameters are the variables declared in the function definition. They act as placeholders for the actual values that will be passed as arguments when the function is called. Formal parameters are typically used within the function to perform certain operations or calculations.
What is the difference between function declaration and definition?
A function declaration is a statement that specifies the function’s name, return type, and parameters. It allows the compiler to recognize the existence of the function. On the other hand, a function definition provides the actual implementation of the function’s behavior. It includes the complete details of the function, such as the code that is executed when the function is called.
What is Call by value and Call by references?
When we pass a value of a variable to a function is called a call by value. And When we pass the address of a variable to a function is called call by references.
|
|
Can we return multiple values in the same function in C?
In C, it is not possible to return multiple values directly from a function. However, there are a few workarounds to achieve this. One common approach is to use pointers as parameters in the function, which allows modifying variables outside of the function’s scope. Another option is to use structures or arrays to encapsulate multiple values and then return or manipulate them as needed. These methods enable the return of multiple values from a function in C.
How many parameters we can use maximum for a single function in C?
The maximum number of parameters that can be used for a single function in the C programming language is not specified. The number of parameters is typically determined by the compiler’s limitations and the platform’s memory constraints.
Can we pass an array to the function as an argument?
Yes, it is possible to pass an array as an argument to a function.
Can we pass a structure to the function as an argument?
Yes, it is possible to pass a structure as an argument to a function.
Can we return a pointer from the function in C?
In C programming, it is possible to return a pointer from a function.
|
|
In our next article, we will discuss about the preprocessor in C programming.
You can also read the below tutorials.
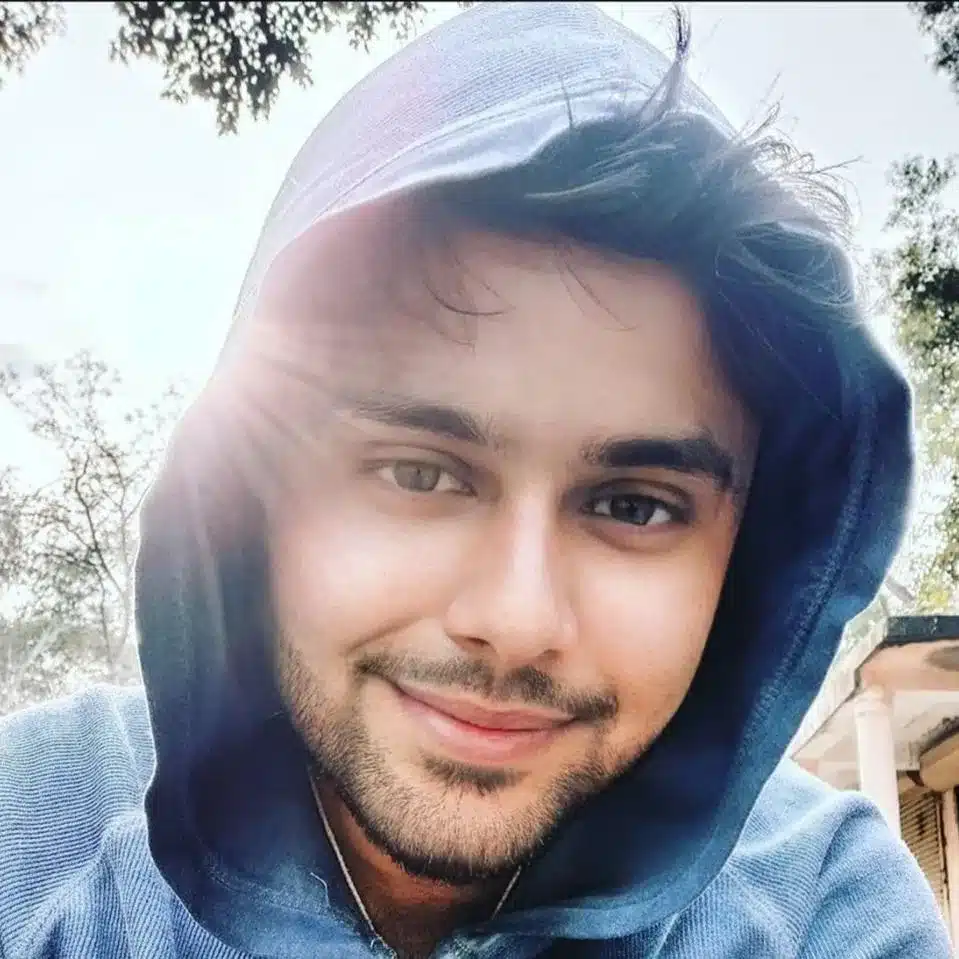
Embedded Engineer|| C programming || Microcontroller
Hi. I am Sunil an Electrical Engineer as well as an Embedded Engineer. I had interested in embedded systems to learn things and to implement my working ideas practically on embedded and all their domains. So here you can find my articles which have been created by me in a simple way to make you understand its concepts. I believe that learning things and after that implementing them practically is a good way to gain experience.
Thank you for reading my articles keep supporting and keep learning!