This article is the continuation of the Series on the C programming tutorial and carries the discussion on C language programming and its implementation. In our last tutorial, we discussed loops in C programming like for loop, while, and do-while loop in C programming. In this article, we are going to see Jump statements in C (break, continue, goto, return in C programming). It aims to provide easy and practical examples for understanding the C program.
Table of Contents
Jump Statements in C
Jump statements in C are used to alter the normal sequence of execution of a program. They allow the program to transfer control to a different part of the code.
Types of Jump Statements in C
There are four types of jump statements in C,
What is Break Statement?
The break
statement is used to terminate the loop or switch statement it is contained within. It allows the program to exit the loop or switch and continue executing the next statement after the loop or switch. The break statement shall appear only in the “switch” or “loop”. The statements appearing after the break
in the loop will be skipped.
Note: If we use the break statement inside of a nested loop, the break statement will first break the inner loop. So, it won’t break all the loops.
|
|
Syntax
do { //statement(s) break; } while (condition);
How break statement work?
Check the below image. In that, we are breaking the for loop
if certain conditions are met in the if
condition. So, the for loop
will be running continuously until two cases. Either the condition in the for loop should fail or the condition in the if should pass.
Example Source Code
#include <stdio.h> int main() { int i; double num, sum = 0.0; for (i = 1; i <= 10; ++i) { printf("Enter n%d: ", i); scanf("%lf", &num); // if the user enters a negative number, break the loop if (num < 0.0) { break; } sum += num; // sum = sum + num; } printf ("Sum = %.2lf", sum); return 0; }
Output
This above program calculates the sum of a maximum of 10 numbers. Why a maximum of 10 numbers? It is just an example to explain the break
statement. If the user enters a negative number anytime, the break
statement is executed in the for loop. This will end that for loop
, and the sum is displayed. If there is no negative number provided by the user, the for loop will be executed 10 times.
The below output shows when the user enters one negative value after 3rd iteration. So, it won’t execute 4th iteration.
Enter n1: 2.4 Enter n2: 4.5 Enter n3: 3.4 Enter n4: -3 Sum = 10.30
The below output shows when the user doesn’t enter a negative value. So, it won’t execute all 10 iterations.
Enter n1: 1.1 Enter n2: 2 Enter n3: 3.1 Enter n4: 4 Enter n5: 5 Enter n6: 6.1 Enter n7: 7.1 Enter n8: 8.1 Enter n9: 9.2 Enter n10: 10.1 Sum = 55.80
What is Continue Statement?
The continue
statement is used to skip the remaining statement(s) in the current iteration of a loop and move to the next iteration. It allows the program to jump to the next iteration without executing the statements below the continue
statement.
|
|
Syntax
do { if(condition1) { //Statements 1 continue; } //Statements 2 } while (condition);
How does the continue statement work?
Check the below image. In that for
loop, we have used the continue
statement when the condition1 is evaluated as true
. So, the statement1 and statemen2 will be skipped only for this iteration, and it continuous to the next iteration. This continue
statement won’t stop the loop like a break
statement.
Example Source Code
#include <stdio.h> int main() { int i; double num, sum = 0.0; for (i = 1; i <= 10; ++i) { printf("Enter a n%d: ", i); scanf("%lf", &num); if (num < 0.0) { continue; } sum += num; } printf("Sum = %.2lf", sum); return 0; }
Output
The above program calculates the sum of the input values. If the user enters any negative value, then it won’t do an addition operation for this iteration as we have used continue
statement. So it will skip the current iteration, don’t execute any statements under that continue, and go to the next iteration. The below output explains that. When the user enters a positive number, the sum is calculated using sum += number. When the user enters a negative number, the continue statement is executed and it skips the negative number from the calculation. We have entered negative values at the 2nd, 5th, and 10th iterations. But these values are not added into the sum
.
Enter a n1: 1 Enter a n2: -5 Enter a n3: 2 Enter a n4: 3 Enter a n5: -4 Enter a n6: 7 Enter a n7: 8 Enter a n8: 9 Enter a n9: 10 Enter a n10: -105 Sum = 40.00
What is the goto statement?
The goto statement is one of the Jump statements in C. That is used to transfer control directly to a labeled statement in the program. It allows the program to jump to a different part of the code, skipping any statements in between. The label in the goto statement is a name used to direct the branch to a specified point in the program. A goto statement causes an unconditional jump to a label statement.
Note: It is important to use jump statements judiciously, as they can make the code less readable and harder to maintain and, it sometimes becomes tough to trace the flow of the code. They should be used sparingly and only when necessary for control flow purposes. So, this goto statement will be avoided in general programming.
Syntax
goto Label; . . Label: { statement n; }
Example Source Code
#include <stdio.h> int main() { int num; printf("Enter a positive or negative integer number: "); scanf("%d",&num); if(num >= 0) { goto pos; } else { goto neg; } pos: printf("%d is a positive number",num); return 0; neg: printf("%d is a negative number",num); return 0; }
Output
In the above program, we are jumping to the respective label based on the user input. So, it won’t execute the intermediate statements before that label.
|
|
- If the user has entered a positive number, the control is passed to
pos
by goto statement. - If the user entered a negative number, the control is passed to
neg
by goto statement.
Enter a positive or negative integer number: 2 2 is a positive number
This goto statement is used to jump forward or backward. The below example shows how the goto
statement is used to jump backward.
#include <stdio.h> int main() { int num, retry = 3; RETRY: if(retry == 0) { return 0; } printf("Testing goto backward - Retry = %d\n", retry); retry--; goto RETRY; }
The output of the above program is given below. The program executes 3 times as the retry value is 3. In that, we have used the goto statement at the bottom of the code and the label is at the top of the code.
Testing goto backward - Retry = 3 Testing goto backward - Retry = 2 Testing goto backward - Retry = 1
What is the return statement in C?
The return
statement in the C programming language is used to end the execution of a function and returns a value to the caller of the function. It is especially useful when you want to pass a result back to the code that called the function. The return
statement is mostly used at the end of a function. You can also add a return keyword at any place in the function. But it is not advisable and it would be better to use that in the end. By default, functions do not return a value.
The function may return the below things.
Also, the function returns the value that belongs to the same data type as it is declared.
|
|
Syntax
return <value or expression>;
Example
We will explain the return using the below program.
#include <stdio.h> int add(int a, int b) { return ( a + b); } int main() { int sum, a, b; printf("Enter a: "); scanf("%d", &a); printf("Enter b: "); scanf("%d", &b); sum = add(a, b); printf("The sum is : %d", sum); return 0; }
Output
In the above program, we are getting two values called a and b. Then we are passing that values to the function called add()
. If you closely look at the function add()
, it has a return
statement with the addition of a
and b
. That function returns that value and it is stored in the variable called sum
. So, the value is returned to the variable sum
.
Enter a: 1 Enter b: 5 The sum is : 6
Note: If you forget to return a value in a function that is defined with the return type, most of the C compilers will throw you a warning. And the return value should match the data type that is being declared with that function.
Frequently Asked Question
Can we use break anywhere other than loops in C?
Yes, in the C programming language, the keyword break
is commonly used to terminate loops, such as for
, while
, and do-while
loops. However, break
can also be used to exit from a switch
statement, which is not a loop construct. So, in addition to loops, break
can be used in C to break out of a switch case.
If I use the break in the nested loop, will that break all the loops in C?
In the C programming language, using the break
statement in a nested loop will only terminate the innermost loop, not all the loops. For example, consider the below program.
|
|
#include <stdio.h> int main() { for(int i=0; i<=1; i++) // outer loop { for(int j=1 ;j<=10; j++) // inner loop { if(j == 5) { break; } } } }
In the above program, there are two for
loops (outer and inner). And when the j
value is equal to 5, we are using the break statement that exits only the inner loop, not the outer loop.
Can I use both the continue and break statements in the same loop in C?
Yes, you can use both the continue
and break
statements in the same loop in C. The continue
statement is used to skip the remaining statements in the loop and move to the next iteration, while the break
statement is used to exit the loop entirely.
Can we jump from one function to another function using the goto statement in C?
In C, it is not possible to jump from one function to another using the `goto
` statement. Because labels are local to that single function. You will get an error called “error: label ‘LABEL_NAME’ used but not defined“.
Can we use multiple return keywords in the same function in C?
In C, it is possible to use multiple return keywords in the same function. But if one return keyword got executed, then other statements that are present under that return keyword won’t get executed. Check the below example program.
#include <stdio.h> int main() { int num; printf("Enter a number: "); scanf("%d", &num); if( num == 0 ) { printf("%d is a equal to Zero\n",num); return 0; } else if( num > 0 ) { printf("%d is a Positive number.\n",num); return 1; } else { printf("%d is a Negative number.\n",num); return -1; } return 0; }
In the above program, we are returning from 3 places.
|
|
Can we return any value from the function with a void return type?
No, we can just use keyword return without any value or expression. A function with a void return type does not explicitly return a value. Instead, it is used to indicate that the function does not need to return any value.
Can we return the integer from the function with the char return type?
No, it is not possible to return an integer from a function with a char return type. The compiler will throw you a warning.
In our next tutorial, we will see the Arrays in C programming.
You can also read the below tutorials.
|
|
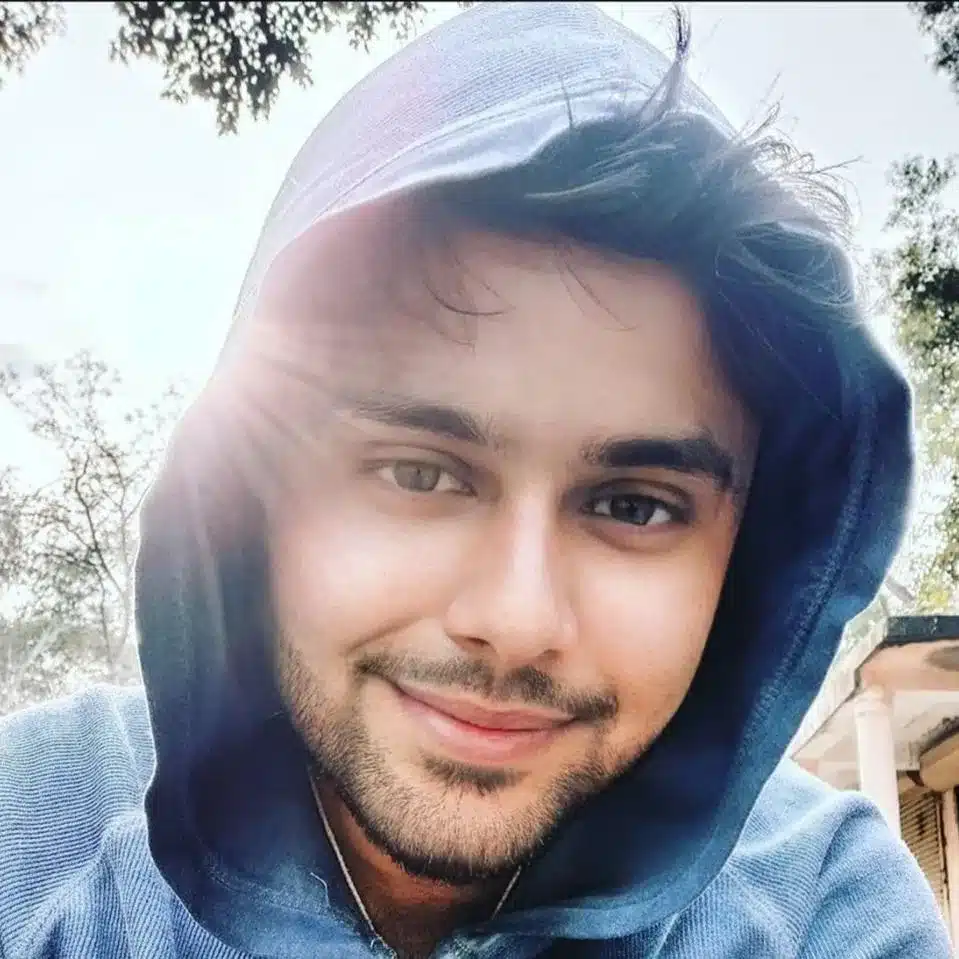
Embedded Engineer|| C programming || Microcontroller
Hi. I am Sunil an Electrical Engineer as well as an Embedded Engineer. I had interested in embedded systems to learn things and to implement my working ideas practically on embedded and all their domains. So here you can find my articles which have been created by me in a simple way to make you understand its concepts. I believe that learning things and after that implementing them practically is a good way to gain experience.
Thank you for reading my articles keep supporting and keep learning!