This article is the continuation of the Series on the C programming tutorial and carries the discussion on C language programming and its implementation. In our last tutorial, we discussed conditional statements like if, if…else, else-if, and switch cases in C programming. In this article, we are going to see one of the flow control statements that are loops in C (For, While, Do While looping control statements in C programming). It aims to provide easy and practical examples for understanding the C program.
Table of Contents
Looping control statements
Prerequisites
We would like to recommend that you read the following articles for a better understanding of the topic at hand. It is highly beneficial to familiarize yourself with these additional resources.
What are loops in C programming?
A loop in C programming is a control structure that allows the repetitive execution of a block of statements. It enables a program to perform a certain task multiple times without the need to write the same code repeatedly. There are various types of loops available in C programming, Loops are commonly used in C programming to iterate over arrays, perform calculations, and implement iterative algorithms. Looping control statements are used to execute a particular set of instructions repeatedly until the condition is met or for a fixed number of looping.
Types of loops in C programming
There are three types of loops commonly used in C programming:
What is a while loop in C programming?
A while loop is a control structure in the C programming language. It allows a block of code to be executed repeatedly as long as a specified condition is true. The code within the loop will continue to execute until the condition becomes false.
|
|
A while loop is also known as an entry loop because, in a while loop, the condition is tested first then the statements of the while loop will be executed. If the while loop condition is false for the first time then the statements under the while loop will not be executed.
Syntax
while (condition) // (Evaluated before each execution of loop body) { statement(s); }
Flow chart
How does a while loop work?
- The while loop runs the condition inside the parentheses ().
- If the condition is true, statements inside the body of the while loop will be executed. Then, the condition will run again. The process will continue until the condition is true.
- If the condition is false, then the loop will terminate (end).
Example source code
#include <stdio.h> int main() { int i = 1; while (i <= 5) { printf("%d\n", i); ++i; } return 0; }
Output
In the above program, we have initialized i
to 1. When i = 1, the condition i <= 5
is true. Then, the body of the while loop will be executed. It prints 1 on the screen and the value of i is incremented by 1. Now, the value of the i = 2. The condition i <= 5
is again true. Then The body of the while loop is executed again. It prints 2 on the screen and the value of i
is incremented to 3. This process goes on until when i
become 6. When the value of i
is 6, the condition i <= 5
will be false and then the loop terminates.
1 2 3 4 5
What is do while loop in C programming?
A do-while loop in C programming is a control flow statement that executes a block of code repeatedly as long as a specified condition is true. Unlike a regular while loop, which checks the condition before the code block is executed, a do-while loop executes the code block first and then checks the condition. This guarantees that the code block is executed at least once, even if the condition is initially false.
Do-while loop is known as exit loop because as we mentioned earlier, the statements will be executed first and then the condition will be checked.
Syntax
do { statement(s); } while (condition); //(Evaluated after each execution of loop body)
Flow chart
How does the do-while loop work?
- The statements which are inside the do{} will be executed.
- Then it will check the condition.
- If the condition is true, then the body of the do-while loop will execute again.
- This process goes on until the condition becomes false.
- If the condition is false, then the loop gets end.
Example
#include <stdio.h> int main() { double number, sum = 0; // the body of the loop is executed at least once do { printf("Enter a number: "); scanf("%lf", &number); sum += number; } while(number != 0.0); printf("Sum = %.2lf",sum); return 0; }
Output
In the above, we have used a do-while loop for the user to enter a number. The loop works as long as the input number is not 0. The do-while loop executes at least once i.e. the first iteration runs without checking the condition. The condition is checked only after the first iteration has been executed.
|
|
If the first input is a non-zero number, then that number is added to the variable sum
and the loop continues to the next iteration. This process is repeated until the user enters 0. But if the first input is 0, there will terminate the loop and prints the value of sum
variable.
Enter a number: 1.5 Enter a number: 2.4 Enter a number: -3.4 Enter a number: 4.2 Enter a number: 0 Sum = 4.70
What is a for loop in C programming?
A for loop in C programming is a control flow statement that allows repetitive execution of a block of code. It consists of an initialization expression, a condition expression, an increment or decrement expression, and a statement or block of statements. The initialization expression is executed once at the beginning of the loop, the condition expression is evaluated before each iteration to determine whether the loop should continue or not, and the increment or decrement expression is executed after each iteration (after executing the statements in the for loop). If the condition expression evaluates to true, the statement or block of statements is executed, and then the increment or decrement expression is evaluated. This process continues until the condition expression evaluates to false. The for loop is commonly used for iterating over a specific range of values or for performing a task a specific number of times.
Syntax
for ( initialization; condition; incrementation or decrementation ) { //statement(s); }
Flow chart
How does for loop work in the C program?
The initialization statement is executed only once and that too in the very first time. Then, the condition is evaluated. If the test condition is evaluated as false, then for loop will be terminated. If the test condition is evaluated as true, then the statements inside the body of for loop will be executed, and after that incrementation or decrementation will happen. Then again the test condition will be evaluated. This process goes on until the test condition is false. When the test condition is false, the loop terminates.
Example
#include <stdio.h> int main() { int i; for( i = 1; i < 11; ++i ) { printf("%d ", i); } return 0; }
Output
Initially, i
is initialized to 1. The test condition i < 11
is evaluated. Since 1 is less than 11 and the condition is true, the body of for loop is executed. This will print the 1 (value of i) on the screen. The update statement ++i
is pre-increment. Now, the value of i
will be 2. Again, the test condition is evaluated as true, and the body of for loop is executed. This will print 2 (value of i) on the screen. Again, the update statement ++i
is pre-increment, and the test condition i < 11
is evaluated. This process goes on until i
become 11. When i
becomes 11, i < 11
will be false, and for loop terminates.
1 2 3 4 5 6 7 8 9 10
Frequently Asked Questions
Why do we need loops in C program?
Loops are an essential part of C programming as they help in repeating a certain block of code multiple times. These repetitions are particularly useful when performing tasks such as iterating through elements in an array, implementing conditional statements, or executing a set of instructions until a certain condition is met. By using loops, C programmers can write efficient and concise code that saves time and effort in executing repetitive tasks.
|
|
What are the applications of loops in C program?
The applications of loops in C programming are varied and beneficial. Loops allow for the repetition of code, which can be helpful in performing tasks that require iterating over a set of values or executing a piece of code multiple times. Some common applications of loops in C programs include: –
- Iterating over arrays or collections of items: Loops can be used to access and manipulate each element of an array or a collection, allowing for efficient and organized processing of data.
- Performing calculations or computations: Loops can be used to perform complex calculations or computations by repeating a set of instructions until a specific condition is met.
- Implementing iterative algorithms: Loops are essential for implementing iterative algorithms, such as sorting or searching algorithms, as these algorithms require repetitive operations to achieve the desired result.
- Handling user input validation: Loops can be used to repeatedly prompt the user for input until valid data is provided, ensuring that the program operates with appropriate and expected values. By utilizing loops effectively, C programmers can enhance the functionality and efficiency of their programs.
What is a nested loop in C programming?
A nested loop in C programming refers to a loop that is defined inside another loop. This allows for the execution of a set of statements repeatedly, depending on the conditions specified, within the enclosing loop. The nested loop can be used to perform tasks such as iterating through a two-dimensional array, traversing linked lists, or processing matrices. It provides a way to implement complex patterns of repetition in a program.
What are loops available in the C program?
In the C programming language, there are several types of loops available. These are three types of loops commonly used in C programming:
Can we use multiple conditions in the while loop in the C program?
Yes, you can use multiple conditions in the while loop in a C program. The while loop will continue executing as long as all the conditions are true. We can combine the conditions using AND or OR operators.
Example
|
|
while( condition1 && condition2) { //Statements } while( condition1 || condition2) { //Statements }
What if the condition in the while loop is always true in the C program?
If the condition in the while loop in a C program is always true, it would result in an infinite loop. This means that the loop will continue to execute indefinitely since the condition is never false. This can be problematic because it may lead to the program never reaching its intended termination point. As a result, the program may consume excessive CPU resources and potentially cause the system to become unresponsive. It is essential to ensure that the condition in a while loop eventually becomes false to prevent infinite looping.
But we use the infinite loop in the Embeed system application. The expression “while(1)” is commonly used in the field of embedded systems. It represents an infinite loop, where the code inside the loop will be executed repeatedly as long as the condition “1” remains true. We use that while(1) inside the main function.
Example
#include "LED.h" int main() { while(1) { LED_On(); delay_ms(100); LED_Off(); delay_ms(100); } }
The above code is used to blink the LED continuously.
What is the difference between the while loop and the do-while loop in C program?
The while loop and the do-while loop are both used in C programming but they differ in how they evaluate the condition. In the while loop, the condition is checked before the execution of the loop. If the condition is false initially, the loop will not be executed at all. In contrast, the do-while loop executes the loop first and then checks the condition. This means that the do-while loop will always execute at least once, regardless of the condition being true or false.
|
|
What is the use of do { } while(false) in the C program?
The `do { } while(false)` loop in a C program is primarily used to create a block of code that will always execute just once, regardless of the condition. This can be useful in certain scenarios. Consider the below program.
#include <stdio.h> int main() { if( condition1 ) { return -1; } if( condition2 ) { return -2; } if( condition3 ) { return -3; } return 0; }
In the above program, the main function has multiple if conditions. And if any condition becomes true, it is returning from there. Thus, it has multiple return points. It is not always good practice. It will be difficult to debug the problem if we encounter anything. We should return from only one place, and that too at the last of that function. We can use the do-while (false) for this scenario. Check the below program.
#include <stdio.h> int main() { int ret = 0; do { if( condition1 ) { ret = -1; break; } if( condition2 ) { ret = -2; break; } if( condition3 ) { ret = -3; break; } }while( false ); return ret; }
In the above code, we have only one exit point for the main function. In other places, we have a break statement.
Do we need to have the initialization part always in the for loop in C program?
In C programming, the initialization part is not always required in the for loop. The initialization part is used to initialize the loop control variable, which is commonly used to control the iterations of the loop. However, it is not mandatory to include the initialization part in every for loop. The initialization part can be placed outside the for loop as well, depending on the requirements of the program.
Example
|
|
int i = 0; for( ; i < 10; i++ ) { //statements }
What is for(;;) in the C program?
The for(;;)
in the C programming language is an infinite loop like while(1)
. It is a shorthand way of writing a loop that has no condition to terminate. The loop will continue indefinitely until a break
statement is encountered or the program is interrupted.
In our next article, we will discuss the Control Transfer Statements or Jumping Statements in the C program.
You can also read the below tutorials.
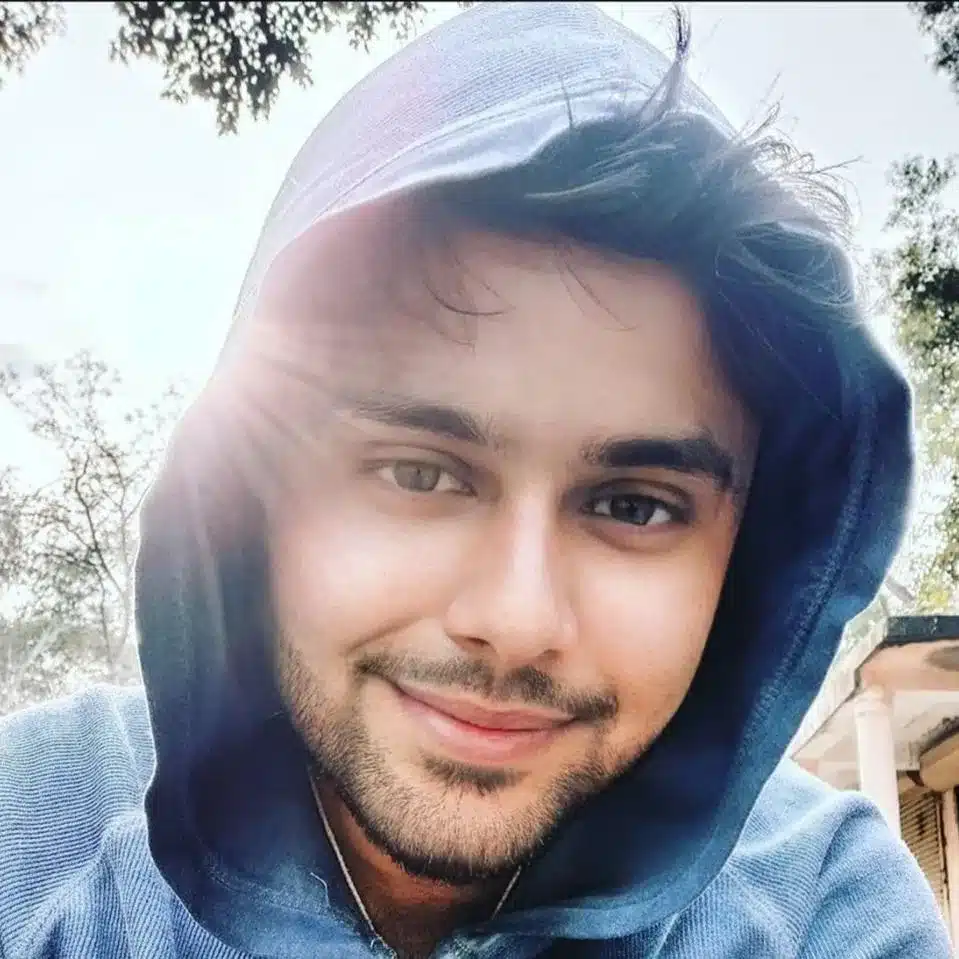
Embedded Engineer|| C programming || Microcontroller
Hi. I am Sunil an Electrical Engineer as well as an Embedded Engineer. I had interested in embedded systems to learn things and to implement my working ideas practically on embedded and all their domains. So here you can find my articles which have been created by me in a simple way to make you understand its concepts. I believe that learning things and after that implementing them practically is a good way to gain experience.
Thank you for reading my articles keep supporting and keep learning!