This article is the continuation of the Series on the C programming tutorial and carries the discussion on C language programming and its implementation. In our last tutorial, we discussed flow control in C programming. Flow control has many control statements. In this article, we are going to see one of the flow control statements that are conditional statements (if…else, else-if, nested if, switch case in C programming). It aims to provide easy and practical examples for understanding the C program.
Table of Contents
Prerequisites
We would like to recommend that you read the following articles for a better understanding of the topic at hand. It is highly beneficial to familiarize yourself with these additional resources.
Recap of our last tutorial
In our last tutorial, we discussed flow control in C programming. Flow control refers to the execution order of statements or instructions in a program. It allows developers or programmers to control the flow and sequence of code execution based on certain conditions or criteria. Flow control statements are used to make decisions, repeat blocks of code, and jump to specific sections of code.
There are several types of flow control statements commonly used in C programming:
- Conditional Statements
- Looping Statements
- Control Transfer Statements
In this article, we will focus on Conditional Statements.
|
|
Conditional Statements in C
Conditional statements in C are used to execute certain blocks of code based on certain conditions. These statements allow the program to make decisions and control the flow of execution. These conditional statements in C are essential in programming, as they allow the program to make decisions and perform actions based on different situations.
Types of Conditional Statements in C
It is classified into five types.
- Simple if statements
- if-else statements
- else-if statements
- Nested if statements
- switch case statements
What is an if statement in C?
The “if
” statement is one of the most common conditional statements in C programming. Simple if statement is used to change the flow and execute some code when the condition is true. It is used to check if a certain condition is true, and if it is true, the code within the block following the “if
” statement will be executed. If the condition is not true (false), the code within the “if
” block will be skipped.
Syntax
if(condition) { /* your code */ }
if statement uses
The very common examples of the if statement are given below.
- Is A bigger than B?
- Is X equal to Y?
- Is M not equal to N?
Flow chart
How if statements work?
- If the test condition is evaluated as true, then the statements inside the body are executed.
- If the test condition is evaluated as false, then the statement inside the body is not executed.
Check the below image. In that, take the first case. The n’s value is 15. And we are checking whether n is less than 20 or not. Yes, it is less than 20 as the value of the n is 15. So, the condition is true. Thus, it will execute the statements inside the if block. In the second case, we are checking whether n is greater than 20 or not. That condition is false. So, it will not execute the statements inside the if block, skips it, and jumps to after the if block.
|
|
Example Source code
#include <stdio.h> int main() { int num; printf("Enter a number: "); scanf("%d", &num); //if the number is less than 0 (true) if (num < 0) { printf("%d is a negative number.\n",num); } printf("Goodbye!!!"); return 0; }
Output
Enter a number: -2 -2 is a negative number. Goodbye!!!
Here users enter -2, and then the test condition num < 0 is evaluated as true. So, it displayed (“You entered -2 is negative“) on the screen because -2 is a negative number. Then it executes the line (Goodbye!!!) that is present after the if condition.
Now, we will enter the positive number.
Enter a number: 5 Goodbye!!!
Here users enter 5, and then the test condition num < 0 is evaluated as false and the statement inside the body will not be executed because 5 is not a negative number. So, it skips the statement inside the if condition and executes the line (Goodbye!!!) that is present after the if condition.
What is the if-else statement in C?
The if-else statement in the C programming language allows you to execute a block of code if a certain condition is true, and another block of code if the condition is false. It provides a way to make decisions and control the flow of the program based on different conditions. This else block is optional.
Syntax
if(condition) { //statement(s) 1; } else { //statement(s) 2; }
Flow chart
How if-else statement works?
- If the test condition is evaluated as true, then the statement inside the body of if is executed. So the statement inside the body of else will be skipped from execution.
- If the test condition is evaluated as false, then the statement inside the body of else is executed. So the statement inside the body of if will be skipped from execution.
Example Source code
#include <stdio.h> int main() { int num; printf ("Enter an number: "); scanf ("%d", &num); //if the remainder is 0 (true) if (num %2 == 0) { printf ("%d is an even number.”,num); } else { printf ("%d is an odd number.”,num); } printf("Goodbye!!!"); return 0; }
Output
Enter an number: 7 7 is an odd number. Goodbye!!!
Here user enters 7 and the test condition num%2==0 is evaluated false. So, the statement inside the body of else will be executed.
|
|
Now we will enter the even number.
Enter an number: 4 4 is an even number. Goodbye!!!
What are else-if statements in C?
Another conditional statements in C is the “else if” statement, which allows for testing multiple conditions. In this case, if the condition of the preceding “if” statement is false, the program checks the condition of the “else if” statement. If it is true, the code within the corresponding block is executed. Lastly, the “else” statement is used as a default option when all the preceding conditions are false. If none of the conditions in the “if” and “else if” statements are true, the code within the “else” block is executed. We can add any number of else-if statements after the if condition.
Syntax
if (condition1) { statement(s) 1; } else if (condition2) { statement(s) 2; } else if (condition3) { statement(s) 3; } else { statement(s) 4; }
Flow chart
How else-if statements work?
Suppose the if condition is true, and then the statements under the first if condition will be executed. If the if condition is false and the next else-if condition is true, then the particular statements under that else-if will be executed. If none of the if and else-if conditions are true, then the else part will be executed.
Example Source code
#include <stdio.h> int main() { int num1, num2; printf("Enter two numbers: "); scanf("%d %d", &num1, &num2); //checks if the two integers are equal. if(num1 == num2) { printf("Result is %d = %d",num1,num2); } //checks if number1 is greater than number2. else if (num1 > num2) { printf("Result is %d > %d", num1, num2); } //checks if both conditions are false else { printf("Result: %d < %d",num1, num2); } return 0; }
Output
Below, we have given three outputs. Just go through it. You will understand it easily.
Output 1
|
|
Enter two numbers: 10 20 Result: 10 < 20
Output 2
Enter two numbers: 10 10 Result is 10 = 10
Output 3
Enter two numbers: 20 12 Result is 20 > 12
What are nested if-else statements in C?
Nested if-else statements in the C programming language refer to using if statements inside another if statement. This allows for more complex decision-making in a C program. In simple terms, a nested if-else statement is an if-else statement inside another if-else statement. It helps programmers to create multiple branches of conditions and execute different blocks of code based on those conditions. By nesting if-else statements, programmers can evaluate multiple conditions without limitations.
Syntax
if (condition 1) { if (condition 2) { //statements 1 } else { //statements 2 } } else { if (condition 3) { //statements 3 } else { //statements 4 } }
Flow chart
How nested if-else work?
The below program relates two integers using either < > and = similar to the if-else program. However, we will use a nested if-else statement to solve the problem.
Example Source code
#include<stdio.h> int main() { int n1, n2, n3; printf("Enter the numbers : "); scanf("%d %d %d", &n1, &n2, &n3); if(n1 > n2) { if(n1 > n3) { printf("[if inside if] %d is greater", n1); } else { printf("[else inside if] %d is greater", n3); } } else { if(n2 > n3) { printf("[if inside else] %d is greater", n2); } else { printf("[else inside else] %d is greater", n3); } } }
Output
Below, we have given multiple possible outputs. Just go through it. You will understand it easily.
|
|
Output 1
Enter the numbers : 33 22 11 [if inside if] 33 is greater
Output 2
Enter the numbers : 33 11 44 [else inside if] 44 is greater
Output 3
Enter the numbers : 10 20 5 [if inside else] 20 is greater
Output 4
Enter the numbers : 11 22 33 [else inside else] 33 is greater
Note: If the body of an if-else statement has only one statement, then it is not required to use curly brackets {}. However, it is considered good practice to include the block within curly brackets to improve code readability and avoid potential errors that may arise from complex conditions or nested statements. But if the if-else statements have more than one line, then we should use the curly brackets ({}).
|
|
Example
if (a > b) { printf ("Hello"); } else { printf("Welcome"); } printf ("Hi");
The above code is equal to the below code.
if (a > b) printf ("Hello"); else printf("Welcome"); printf ("Hi");
What is a switch case in C?
This is one of the conditional statements in C program. A switch case in the C programming language is a control structure that allows the execution of different pieces of code based on the value of a given expression. The switch case statement will allow multi-way of branching. It is often used when there are multiple possible outcomes for a particular situation. Depending on the condition and the control is transferred to the particular case label and the statements under that label are executed. If none of the cases are matched with the switch case condition, then the default statement is executed.
Syntax
switch (selection) { case LABEL1: statement(s) 1; break; case LABEL2: statement(s) 2; break; case LABEL3: statement(s) 3; break; default: statement(s) 4; }
Flow Chart
How does the switch statement work?
The condition will be evaluated once and that will be compared with the values of each case label. If there is a match with any label, then the corresponding statements under that matching label are executed. For example, if the value of the condition is equal to LABEL2, then the statements under the case LABEL2 are executed until a break is encountered. If there is no match, the default statements are executed.
Note:
|
|
- If we do not use the
break
statement in the case label, then it will execute the next case also even if the condition is not matching with the label.
- The default case inside the switch statement is optional.
Example
#include <stdio.h> int main() { int MyChoice; printf("Enter your choice: "); scanf("%d", &MyChoice); switch(MyChoice) { case 1: printf("You entered 1.\n"); break; case 2: printf("You entered 2.\n"); break; case 3: printf("You entered 3.\n"); break; default: printf("Invalid choice.\n"); break; } return 0; }
Output
We have given all the possible outputs of the above example for your understanding.
Output 1
Enter your choice: 1 You entered 1.
Output 2
Enter your choice: 2 You entered 2.
Output 3
Enter your choice: 3 You entered 3.
Output 4
|
|
Enter your choice: 5 Invalid choice.
In the above program, the user is prompted to enter a number of their choice. The switch statement then checks the value of the variable MyChoice
. If the value matches one of the cases (1, 2, or 3 in this example), the corresponding block of code in that respective label will be executed. If the value that the user entered doesn’t match any of the cases in the switch case, the code in the default
block is executed.
Frequently Asked Questions
Is “else” required always in the if statement in the C program?
In a C program, the “else” statement is not always required in an “if” statement. It is optional and can be omitted if there is no specific action to be taken when the condition in the “if” statement evaluates to false.
Is it mandatory to use the curly brackets for the if condition block in C programming?
In C programming, it is not mandatory to use curly brackets (flower brackets) for the if condition block if that has only one line. However, it is considered good practice to include the block within curly brackets to improve code readability and avoid potential errors that may arise from complex conditions or nested statements. If the if
block has more than one line, then we should use curly brackets (flower brackets).
What is the meaning of if(1)?
The above statement if(1)
evaluates always True. Any non-zero value will be considered as true and zero (0) is considered false in C. So, if(10)
also evaluates to be true. if(0)
evaluates to be false.
Can we add multiple conditions in the if?
Yes, it is possible to add multiple conditions in an if statement. By using logical operators such as “AND” or “OR”, you can combine multiple conditions within the parentheses of an if statement. This allows the program to execute a specific block of code only if all the conditions are true or at least one condition is true, depending on the logical operator used.
|
|
Example
if( (condition 1) || ( condition 2) ) { // statements } if( (condition 1) && ( condition 2) ) { // statements }
How many else-if we can use in C programming?
In C programming, there is no specific limit on the number of “else-if” statements that can be used. You can use as many “else-if” statements as necessary to create the desired logic in your program.
Is “else” required always in the else-if statement in the C program?
An “else” statement is not always required in an “else-if” statement in a C program. This statement allows the program to make multiple conditional checks, where each condition is checked in order. If none of the conditions are true, the program will skip all the “else-if” blocks and proceed to the code following the last “else” or “else-if” statement. Therefore, including an “else” statement is optional, depending on the desired program logic.
Do we need to add the break in all the cases in the switch statement in the C program?
In C programming, it is not necessary to include a break statement in every case of a switch statement. The break statement is used to exit the switch statement once a match is found. If there is no break statement, the code will continue executing the statements in subsequent cases until a break statement is encountered or the switch statement is completed.
For your understanding, I have removed the break in all the cases. The program is given below.
|
|
#include <stdio.h> int main() { int MyChoice; printf("Enter your choice: "); scanf("%d", &MyChoice); switch(MyChoice) { case 1: printf("You entered 1.\n"); case 2: printf("You entered 2.\n"); case 3: printf("You entered 3.\n"); break; default: printf("Invalid choice.\n"); break; } return 0; }
Now, if I enter the value as 1, it will jump to case 1 and print “You entered 1.” Then there is no break statement after that. So, it will execute the statements inside case 2 also. Then case 2 also doesn’t have a break statement. So, it will execute case 3 as well. Since case 3 has a break statement, it will exit from there. For your understanding, we have given the possible outputs of the above program.
Output 1
Enter your choice: 1 You entered 1. You entered 2. You entered 3.
Output 2
Enter your choice: 2 You entered 2. You entered 3.
Output 3
Enter your choice: 3 You entered 3.
Output 4
|
|
Enter your choice: 5 Invalid choice.
Do we need to add a default condition in the switch case in C programming always?
In C programming, it is not always necessary to include a default condition in a switch case statement. The default condition is used to handle cases that do not match any of the specified cases. Including a default condition allows the program to execute a specific set of instructions when none of the other cases are satisfied. However, it is up to the programmer to decide whether or not to include a default condition based on the requirements of the program.
Like nested if, can we use nested switch case in C programming?
In C programming, just like with nested if statements, it is possible to use nested switch cases. This means that within a switch case statement, you can have another switch case statement. This can be useful in situations where you need to evaluate multiple conditions and execute different code blocks based on the values of multiple variables. By nesting switch cases, you can create a more comprehensive and flexible decision-making structure within your program.
Can we replace else-if with the switch cases in C programming?
You might have this question as both else-if and switch cases are working in a similar way. Switch cases can be used as an alternative to else-if statements in C programming. However, it is important to note that there are certain limitations and considerations when using switch cases. Switch cases provide a more efficient way to handle multiple choices and can result in cleaner and more organized code. However, they are not always suitable for all scenarios and may have some restrictions. It is recommended to carefully evaluate the requirements of the program before deciding whether to use switch cases or else-if statements.
Which is best? if or switch in C programming?
When comparing the performance of “if
” and “switch
” statements in C programming, it is difficult to determine which one is definitively better. Both constructs have their own advantages and disadvantages, and the choice depends on the specific requirements of the program. The “if
” statement is more flexible and can handle complex conditions by using logical operators. It is generally recommended for making decisions based on multiple conditions or for evaluating logical expressions. However, using nested “if
” statements can quickly make the code complex and difficult to read. On the other hand, the “switch
” statement is useful when comparing a variable against multiple constant values. It provides a more concise and readable way of handling multiple cases. However, the “switch
” statement is limited to checking equality and cannot handle complex conditions. Ultimately, the choice between “if
” and “switch
” statements in C programming depends on the specific situation and the programmer’s preference. It is recommended to consider the readability, maintainability, and efficiency of the code when making a decision.
In our next tutorial, we will see the looping statements in C programming.
|
|
You can also read the below tutorials.
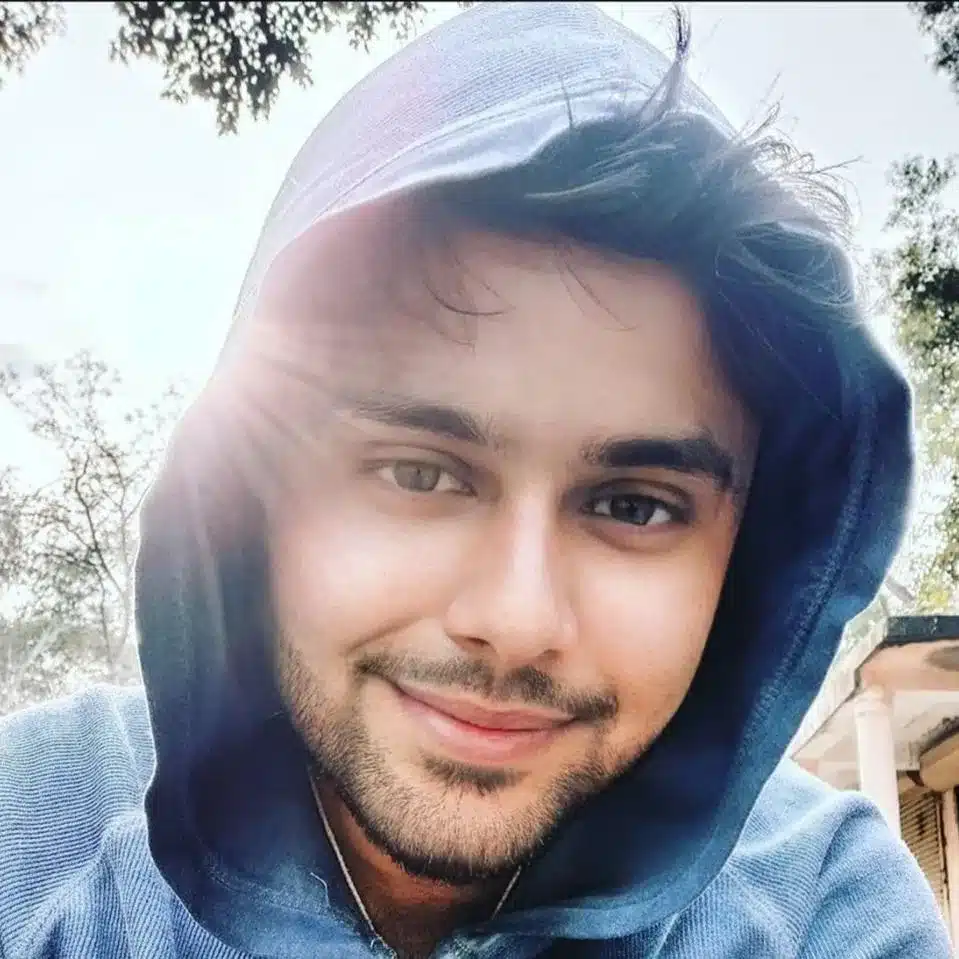
Embedded Engineer|| C programming || Microcontroller
Hi. I am Sunil an Electrical Engineer as well as an Embedded Engineer. I had interested in embedded systems to learn things and to implement my working ideas practically on embedded and all their domains. So here you can find my articles which have been created by me in a simple way to make you understand its concepts. I believe that learning things and after that implementing them practically is a good way to gain experience.
Thank you for reading my articles keep supporting and keep learning!