This article is the continuation of the Series on the C programming tutorial and carries the discussion on C language programming and its implementation. In our last tutorial, we have seen Datatypes in C programming. In this article, we are explaining one of the basic concepts of the C program which is variables in C Language. It aims to provide easy and practical examples for understanding the C program.
When we want to learn C language programming, we need to start from the very basics. First, we need to understand the variables of the C language.
Table of Contents
What are Variables in C programming?
In C programming, variables are used to store data temporarily. They are like containers that hold different types of information such as numbers, characters, or arrays.
A variable is a user-defined custom name assigned to a memory location that helps to store some data or values, like numbers, characters, etc. But you cannot declare a keyword name as a variable name. Variables in C are declared with a specific data type. It can store different types of data in the variable based on the data type and its value can be changed throughout the program, and also it can be reused the same variable many times during the program execution. The size of the variable depends upon the data type which it stores. Each variable has a type, which tells the compiler how big the region of memory is and how to treat the bits which is stored in that region while performing various kinds of operations. If you go through this article, you will come to know the sizes of each data type and how the data is stored in the memory or variable.
Syntax
|
|
datatype variable_name = value;
Rules of Variables
- A variable name only contains alphabets, digits & underscore.
- A variable name should start with either underscore or alphabets.
- A variable name should not same as any keyword’s name.
- Special characters and spaces are not allowed within the variable name.
You should always follow the above rules while doing the programming.
Valid Variables | Invalid Variables |
int name; |
int 2; |
char a = 'c'; |
char goto; |
float sum_result; |
double x y; |
Note: You should always give meaningful names to the variables while doing the programming. That will help to improve the readability of the program.
Example
#include <stdio.h> int main() { int sum = 5; //here sum is a variable and 5 is the initial value. printf("Value is %d", sum); return 0; }
Output
Value is 5
Like this, we can create any number of variables using the proper data types.
|
|
Types of Variables in C Programming
Normally, we can classify the variables into two types.
Let’s see the details one by one.
Local Variables
The variable which is declared inside a block is known as a local variable. The scope of a variable is available within a block in which they are declared. Variables can be accessed only by those statements inside a block in which they are declared. The lifetime of a variable is created when the block is entered and destroyed on its exit. Variables are stored in a stack unless specified.
Example 1
#include <stdio.h> int main() { int a=5; // local variable printf("Value of A = %d",a); return 0; }
In the above program, int a
is defined in the main function. So, it is a local variable to the main
function. We cannot access this variable a
from outside of the main
function.
Example 2
#include <stdio.h> int main() { int a=5; // local variable, can be accessed only from main function if( a == 5 ) { int b = 10; // local variable, can be accessed only from if condition block b = 12; printf("Value of B = %d\n",b); printf("(Inside If) Value of A = %d\n",a); } //here we cannot acceess the variable b printf("Value of A = %d\n",a); return 0; }
In the above program, int a
is defined in the main function. So, it is a local variable to the main
function. We cannot access this variable a
from outside of the main
function. And int b
is defined inside the if()
condition. So, we can only access it from inside that if()
.
|
|
Output
Value of B = 12 (Inside If) Value of A = 5 Value of A = 5
Example 3
#include <stdio.h> int main() { int a=5; // local variable, can be accessed only from main function if( a == 5 ) { int a = 10; // local variable with same name, can be accessed only from if condition block printf("(Inside If) Value of A = %d\n",a); } printf("Value of A = %d\n",a); return 0; }
In the above program, int a
is defined in the main function. So, it is a local variable to the main
function. We cannot access this variable a
from outside of the main
function. And another int a
is defined inside the if()
condition. In this case, if()
will be using the variable which is defined inside the if()
. It can’t able to access the int a
which is defined in the main
function. Because the compiler will give priority to the recently created local variable.
Output
(Inside If) Value of A = 10 Value of A = 5
Global Variables
In the C programming language, global variables are variables that can be accessed and modified by any function or code block within a program. They are declared outside of any function, typically at the top of a file, and have a global scope. This means that they can be used throughout the entire program. However, it is important to note that the use of global variables should be done with caution, as they can lead to potential issues such as naming conflicts and difficulties in tracking variable changes.
Example 1
#include <stdio.h> int a = 10; // global variable int fun() { printf("(func)Value = %d\n", a); } int main() { fun(); printf("(main)Value = %d\n", a); return 0; }
In the above program, we have created the global variable ‘a
‘. This can be accessed and modified anywhere in the program. If we want to access it from another file within the same program, then we need to use the extern keyword.
|
|
(func)Value = 10 (main)Value = 10
Frequently Asked Questions
Can we modify the value of the variable any number of times in the C program?
Yes, the value of a variable can be modified multiple times in a C program. See the below program for your reference.
#include <stdio.h> int main() { int value = 5; value = 10; value = 20; value = 3; printf("Value is %d", sum); return 0; }
In the above program, we are changing the value of the variable multiple times. So, the output will be “Value is 3“.
Is the initial value required always while creating the variables in C?
When creating variables in the C programming language, the initial value is not always required.
What is the initial value of the variable if the variable doesn’t have any initial value at the time of declaration?
If a variable does not have an initial value at the time of declaration and that is a local variable, it will have an undefined initial value (Garbage value). If a variable does not have an initial value at the time of declaration and that is a global variable, it will have zero (0).
Can we create multiple local variables with the same name in C?
No, in the C programming language, you cannot create multiple local variables with the same name within the same scope. Each variable within a scope must have a unique name to avoid ambiguity and ensure correct program execution. If you try to declare multiple variables with the same name in the same scope, it will result in a compilation error. It is important to choose meaningful and distinctive names for variables to make your code more readable and maintainable.
|
|
Can we create a local variable and a global variable with the same name in C?
Yes, it is possible to create a local variable and a global variable with the same name in the C programming language. However, it is not recommended to do so as it can lead to confusion and make the code difficult to understand and maintain.
Example
#include <stdio.h> int a = 10; // global variable int main() { int a = 15; //local variable printf("(main)Value = %d\n", a); return 0; }
Can I assign float values to int data type variables in C?
Yes, you can assign float values to int data type variables in C. However, the float value will be implicitly converted to an integer. The decimal part of the float value will be truncated, meaning it will be rounded down to the nearest whole number. For example, if you assign the float value 3.9 to an int variable, the value stored in the variable will be 3. But is not advised to do that. Some compilers will throw you a warning. You need to typecast it. It is always advisable to assign the same data type value to the same data type variable.
In modern programming languages, a variable also has a scope (a limit on where the value of the variable can be accessed, and which allows the same name to be used for different variables in different parts of the program) and life (the duration of the variables exist controlling when the program allocates and deallocates space for it). We will see the Storage Classes in C in our next tutorial.
You can also read the below tutorials.
|
|
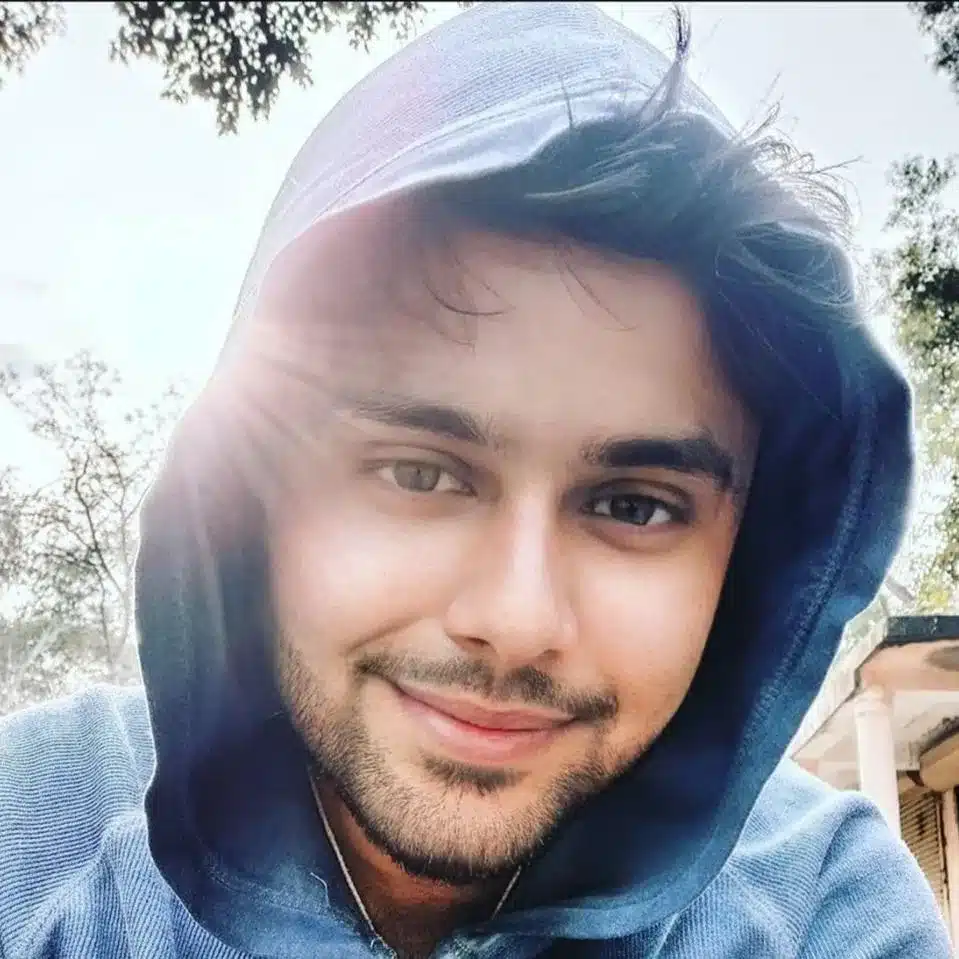
Embedded Engineer|| C programming || Microcontroller
Hi. I am Sunil an Electrical Engineer as well as an Embedded Engineer. I had interested in embedded systems to learn things and to implement my working ideas practically on embedded and all their domains. So here you can find my articles which have been created by me in a simple way to make you understand its concepts. I believe that learning things and after that implementing them practically is a good way to gain experience.
Thank you for reading my articles keep supporting and keep learning!