This article is the continuation of the Series on the C programming tutorial and carries the discussion on C language programming and its implementation. It aims to provide easy and practical examples for understanding the C program. In our last article, we have seen the Structures in C Programming. In this article, we will see Union in C programming. This article is checked by an AI text detector for your trust.
Table of Contents
What is a Union in C Programming?
A union in C programming is a user-defined data type that allows storing different data types in the same memory location. Unlike structures, where each member has its own memory space, all members of a union share the same memory space. The size of a union is determined by the largest member it contains. You can define a union with many members with different data types but only one member can contain a value at a time and the rest of the value will contain garbage value because all union elements share the same memory location for all members.
Syntax
union union_name { datatype 1; datatype 2; };
Unions are commonly used when there is a need to store different types of data in a specific memory location. They are especially useful in scenarios where you need to save memory or when different types of data need to be accessed interchangeably. However, it’s important to note that only one member of a union can be accessed at a time. The value of one member can overwrite the value of another member, leading to unpredictable behavior if the union is not used carefully.
Here’s an example of a union in C:
|
|
#include <stdio.h> union Number { int x; float y; char z; }; int main() { union Number num; num.x = 10; printf("Value of x: %d\n", num.x); num.y = 3.14; printf("Value of y: %.2f\n", num.y); num.z = 'A'; printf("Value of z: %c\n", num.z); return 0; }
In this example, the union Number
can hold an integer, a float, or a character. By assigning values to different members of the union, we can access and print their values accordingly. Keep in mind that changing the value of one member can affect the values of other members due to the shared memory space.
What is the size of the union?
union var { int id; char a; char b; char c; char d; };
The size of the union is 4 bytes
not 8 bytes. If that is the structure, then the size of this is 8 bytes. Because each member will be sharing the different memory spaces. But in the Union, all the members will be sharing the same memory space. So, the size of the union is determined by the size of the largest member. In this case, the largest member is int id
. That’s why the size of this union is 4 bytes.
How to Access Union Members?
You can access the union members by using the ( . ) dot operator which is similar to structures.
var1.member1;
Here var1
is the union variable and member1
is the member of the union.
Example
|
|
#include <stdio.h> union student { int rollNo; char name[32]; double marks; }; union student s; int main() { printf("Size of the Union = %d bytes\n", sizeof(union student)); printf("Enter Name:- "); scanf("%s",s.name); printf("Student name is %s\n",s.name); printf("Enter Roll no:- "); scanf("%d",&s.rollNo); printf("Student roll no is %d\n",s.rollNo); printf("Enter Marks:- "); scanf("%lf",&s.marks); printf("Student percentage is %g\n",s.marks); //Print Other members printf("Student name is %s\n",s.name); printf("Student roll no is %d\n",s.rollNo); return 0; }
Output
Size of the Union = 32 bytes Enter Name:- Sunil Student name is Sunil Enter Roll no:- 1 Student roll no is 1 Enter Marks:- 90.90 Student percentage is 90.9 Student name is ������V@ Student roll no is -1717986918
In the above program, student
is the name of the union. And rollNo
, name
, marks
are members of the union. We can only store data in a single member at the same time, but we can’t store data in multiple union members at the same time. If you see the last two prints (name
and rollNo
), it is a garbage value.
What is the difference between Union and Structure in C?
Union | Structure |
---|---|
Each member has its own separate memory space. | All members share the same memory space. |
The size of the union is determined by the size of its largest member. | The size of the structure is determined by the sum of the sizes of its members. |
Only one member can be accessed at a time. | Individual members can be accessed at a time using the dot operator. |
Only the first member can be initialized explicitly. | All members can be initialized individually or collectively. |
Example:union student |
Example:struct student |
The main differences between unions and structures can be summarized as follows:
- Memory Allocation: Unions have members that share the same memory space, while structures have separate memory space for each member.
- Size: Unions have a size determined by the largest member, while structures have a size determined by the sum of all members’ sizes.
- Member Access: Only one member can be accessed at a time in a union, while structure members can be accessed individually using the dot operator.
- Initialization: Only the first member of a union can be explicitly initialized, whereas all members of a structure can be initialized individually or collectively.
These differences make unions suitable when there is a need to store different types of data in a specific memory location, while structures are commonly used to store related data as separate members.
Frequently Asked Question
Can we add unions in the union in C?
Yes, it is possible to add unions inside a union in C. This concept is known as a nested union in C. By nesting unions, you can further enhance the flexibility and versatility of your data structure.
|
|
Here’s an example:
#include <stdio.h> union Union1 { int a; char b; }; union Union2 { float x; union Union1 nestedUnion; }; int main() { union Union2 u; u.nestedUnion.a = 10; printf("Value of a: %d\n", u.nestedUnion.a); u.x = 3.14; printf("Value of x: %.2f\n", u.x); u.nestedUnion.b = 'A'; printf("Value of b: %c\n", u.nestedUnion.b); return 0; }
In this example, we have a union called Union1
with two members: a
of type int
and b
of type char
. We then have another union called Union2
which contains x
of type float
and a nested union nestedUnion
of type Union1
. By utilizing nested unions, we can store and access different types of data within the same memory location.
Please note that when using nested unions, you need to access the members in a hierarchical manner. For example, in the above code, u.nestedUnion.a
is used to access the a
member of the nested union.
Nested unions can be a powerful tool when working with complex data structures in C, allowing for a flexible arrangement of different types of data. However, it’s important to handle the member access carefully to avoid any unintended behavior.
Can we add unions in the structure in C?
Yes, it is possible to add unions inside a structure in C. By doing so, you can further enhance the flexibility and versatility of your data structure.
|
|
Here’s an example:
#include <stdio.h> struct Employee { int empID; char empName[20]; union { float hourlyRate; int monthlySalary; } payment; }; int main() { struct Employee emp1; emp1.empID = 101; strcpy(emp1.empName, "Sunil"); emp1.payment.hourlyRate = 15.5; printf("Hourly rate: %.2f\n", emp1.payment.hourlyRate); emp1.payment.monthlySalary = 5000; printf("Monthly salary: %d\n", emp1.payment.monthlySalary); return 0; }
In this example, we have a structure called Employee
that contains three members: empID
, empName
, and a nested union payment
. The nested union consists of two members: hourlyRate
and monthlySalary
. Depending on the payment scheme for a particular employee, either the hourly rate or the monthly salary can be accessed and used.
By utilizing nested unions, you can create complex data structures that allow for different types of data to be stored and accessed efficiently. Remember to handle the union members carefully to avoid any unintended behavior.
Can we add structures inside the union in C?
Yes, it is possible to add structures inside a union in C. By nesting structures inside a union, you can create more complex data structures to store and access data.
Here’s an example:
|
|
#include <stdio.h> struct Point { int x; int y; }; union Shape { struct Point point; int radius; }; int main() { union Shape shape; shape.point.x = 10; shape.point.y = 20; printf("Point coordinates: (%d, %d)\n", shape.point.x, shape.point.y); shape.radius = 5; printf("Circle radius: %d\n", shape.radius); return 0; }
In this example, we have a structure called Point
that represents a coordinate point with two integer members: x
and y
. We then have a union called Shape
which can store either a Point
structure or an integer representing the radius of a circle. By utilizing a nested structure inside the union, we can access and manipulate different types of data using the same memory location.
Please note that when using structures inside a union, you need to access the members in a hierarchical manner. For example, in the above code, shape.point.x
is used to access the x
member of the nested structure.
Nested structures inside a union can be useful when you need to store and manipulate different types of data in a flexible manner. However, it’s important to handle the member access carefully to avoid any unintended behavior.
In our next tutorial, we will discuss the pointers in C programming.
You can also read the below tutorials.
|
|
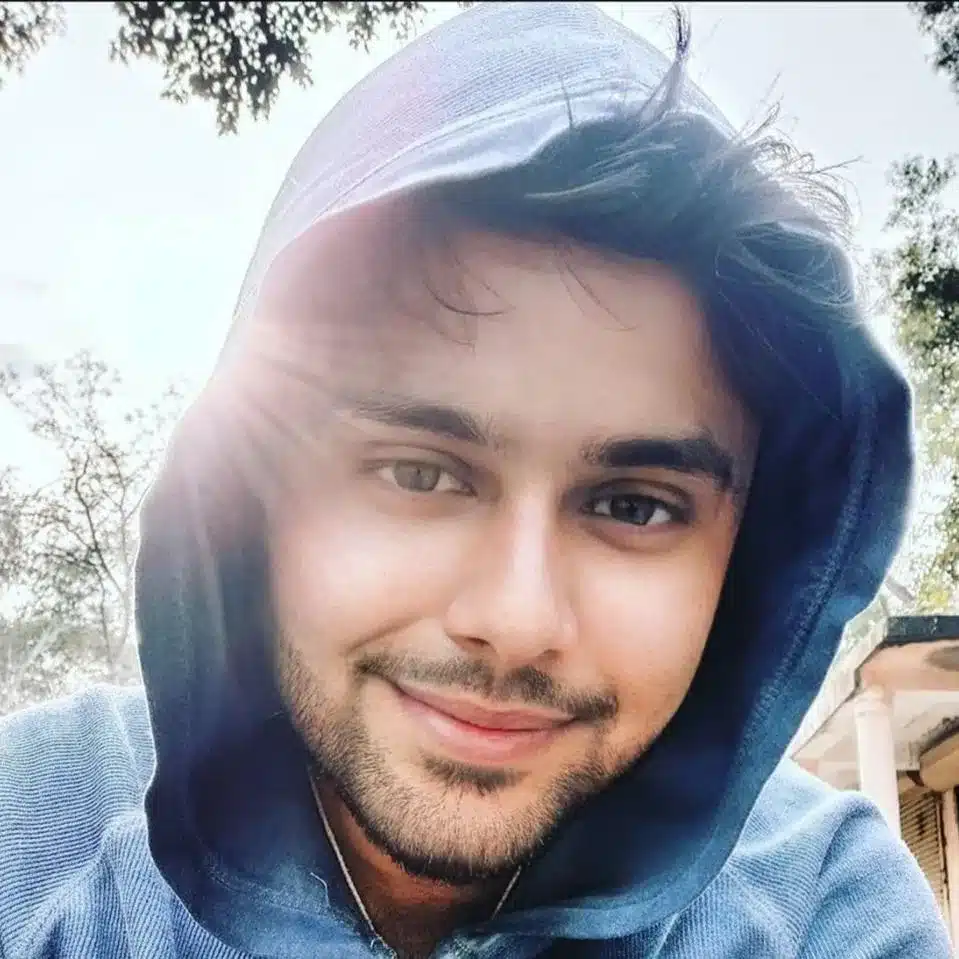
Embedded Engineer|| C programming || Microcontroller
Hi. I am Sunil an Electrical Engineer as well as an Embedded Engineer. I had interested in embedded systems to learn things and to implement my working ideas practically on embedded and all their domains. So here you can find my articles which have been created by me in a simple way to make you understand its concepts. I believe that learning things and after that implementing them practically is a good way to gain experience.
Thank you for reading my articles keep supporting and keep learning!